生成一段基于tensorflow的Mobielnetv3网络分类高光谱图像的代码
时间: 2023-12-10 10:38:43 浏览: 30
以下是一个基于TensorFlow的Mobilenetv3网络分类高光谱图像的代码示例:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, GlobalAveragePooling2D, Dense
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
# 定义输入张量的形状
input_shape = (224, 224, 256)
# 定义输入层
inputs = Input(shape=input_shape)
# 定义卷积层1
x = Conv2D(16, (3, 3), strides=(2, 2), padding='same', activation='relu')(inputs)
# 定义卷积层2
x = bottleneck_block(x, 16, 64, 1, 3)
# 定义卷积层3
x = bottleneck_block(x, 72, 72, 1, 3)
# 定义卷积层4
x = bottleneck_block(x, 72, 72, 1, 5)
# 定义卷积层5
x = bottleneck_block(x, 120, 120, 1, 5)
# 定义卷积层6
x = bottleneck_block(x, 120, 120, 1, 5)
# 定义卷积层7
x = bottleneck_block(x, 240, 240, 2, 5)
# 定义卷积层8
x = bottleneck_block(x, 200, 480, 1, 3)
# 定义卷积层9
x = Conv2D(1024, (1, 1), strides=(1, 1), padding='same', activation='relu')(x)
# 定义全局平均池化层
x = GlobalAveragePooling2D()(x)
# 定义输出层
outputs = Dense(10, activation='softmax')(x)
# 构建模型
model = Model(inputs=inputs, outputs=outputs)
# 编译模型
model.compile(optimizer=Adam(lr=0.001), loss='categorical_crossentropy', metrics=['accuracy'])
```
其中,`bottleneck_block()` 是一个自定义的瓶颈块函数,用于构建Mobilenetv3网络的卷积层。该函数的代码示例如下:
```python
def bottleneck_block(inputs, in_channels, out_channels, strides, expansion_factor):
# 定义扩展层
x = Conv2D(in_channels * expansion_factor, (1, 1), strides=(1, 1), padding='same', activation='relu')(inputs)
# 定义卷积层
x = DepthwiseConv2D((3, 3), strides=strides, padding='same', depth_multiplier=1)(x)
x = BatchNormalization()(x)
x = Activation('relu')(x)
# 定义投影层
x = Conv2D(out_channels, (1, 1), strides=(1, 1), padding='same', activation=None)(x)
x = BatchNormalization()(x)
# 定义残差连接
if strides == 1 and in_channels == out_channels:
x = Add()([x, inputs])
return x
```
请注意,这只是一个示例代码,您需要根据自己的数据集和模型要求进行适当的修改和调整。
相关推荐
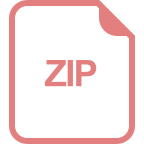
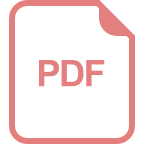
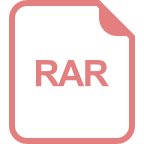














