python读取文件新增内容
时间: 2023-09-23 10:11:30 浏览: 52
要向现有文件中添加新内容,您可以使用打开文件时的追加模式。追加模式将在文件末尾添加新内容,而不会覆盖现有内容。
以下是一个示例代码片段,它打开一个名为“example.txt”的文件,并使用追加模式向其中添加新行。
```python
with open('example.txt', 'a') as f:
f.write('This is a new line\n')
```
这将在“example.txt”文件的末尾添加一行新文本:“This is a new line”。
请注意,打开文件时使用'a'模式,而不是默认的'r'模式。这告诉Python使用追加模式打开文件,而不是读取模式。使用“with”语句的好处是,当代码块完成时,文件将自动关闭。
相关问题
python读取文件新增
您可以使用以下代码读取文件并添加新的内容:
```python
# 打开文件,使用 "a" 模式以添加的方式打开
with open("filename.txt", "a") as myfile:
# 写入新的内容
myfile.write("新的内容\n")
```
以上代码将打开名为 "filename.txt" 的文件,并在文件末尾添加新的内容。请注意,使用 "a" 模式打开文件时,如果文件不存在,则会创建一个新的文件。
Python读取文件夹内新增文件内容上传数据库
可以使用Python的os模块和pymysql模块来实现。首先,使用os模块遍历文件夹内的文件,然后读取新增文件的内容,最后使用pymysql模块将内容上传到数据库中。以下是示例代码:
```python
import os
import pymysql
# 遍历文件夹内的文件
folder_path = '/path/to/folder'
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
if os.path.isfile(file_path):
# 读取新增文件的内容
with open(file_path, 'r') as f:
content = f.read()
# 将内容上传到数据库中
conn = pymysql.connect(host='localhost', user='root', password='password', database='database')
cursor = conn.cursor()
sql = "INSERT INTO table_name (content) VALUES (%s)"
cursor.execute(sql, content)
conn.commit()
cursor.close()
conn.close()
```
注意,需要将`/path/to/folder`替换为实际的文件夹路径,将`localhost`、`root`、`password`、`database`、`table_name`替换为实际的数据库信息。
相关推荐
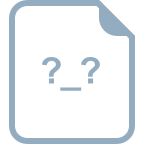
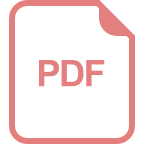












