定义一个顾客类,顾客属性自定义,顾客可以购买食品、玩具两类商品、定义方法输出顾客的信息、购买的商品信息、商品总价值。此外,还可以自定义反映商品折扣情况的方法以及折扣应付金额等信息的输出
时间: 2024-06-09 21:07:25 浏览: 74
以下是一个顾客类的示例代码,包括属性、方法和折扣信息的处理:
```python
class Customer:
def __init__(self, name, age, gender, budget):
self.name = name
self.age = age
self.gender = gender
self.budget = budget
self.items = []
self.total_price = 0
def buy_food(self, food_name, price):
if price <= self.budget:
self.items.append((food_name, price))
self.budget -= price
self.total_price += price
print(f"{self.name}购买了{food_name},价格为{price}元。")
else:
print(f"{self.name}的预算不足,无法购买{food_name}。")
def buy_toy(self, toy_name, price):
if price <= self.budget:
self.items.append((toy_name, price))
self.budget -= price
self.total_price += price
print(f"{self.name}购买了{toy_name},价格为{price}元。")
else:
print(f"{self.name}的预算不足,无法购买{toy_name}。")
def print_info(self):
print(f"顾客姓名:{self.name}")
print(f"顾客年龄:{self.age}")
print(f"顾客性别:{self.gender}")
print(f"购买商品清单:{self.items}")
print(f"商品总价值:{self.total_price}元")
def apply_discount(self, discount_rate):
self.total_price *= discount_rate
print(f"商品打折后总价值:{self.total_price}元")
def print_discount_info(self, discount_rate):
discount_price = self.total_price * (1 - discount_rate)
print(f"商品折扣率为{discount_rate},应付金额为{discount_price}元")
```
在这个类中,初始化方法 `__init__` 会为每个顾客对象设置姓名、年龄、性别和预算等属性,以及一个空列表 `items` 和总价值 `total_price`。`buy_food` 和 `buy_toy` 方法分别用于购买食品和玩具,只有当预算足够时,才能购买成功,并且会更新 `items` 和 `total_price` 属性。`print_info` 方法用于输出顾客的信息、购买的商品清单和总价值。`apply_discount` 方法接受一个折扣率,将总价值乘以该折扣率,然后输出打折后的总价值。`print_discount_info` 方法接受一个折扣率,计算应付的折扣金额,并输出。
以下是一个使用该类的示例:
```python
# 创建两个顾客对象
c1 = Customer("小明", 20, "男", 100)
c2 = Customer("小红", 18, "女", 200)
# 小明购买一些食品和玩具
c1.buy_food("饼干", 10)
c1.buy_toy("小熊", 30)
c1.buy_toy("游戏机", 200)
# 小红购买一些玩具
c2.buy_toy("娃娃", 50)
c2.buy_toy("积木", 30)
# 输出顾客信息和购买清单
c1.print_info()
c2.print_info()
# 小明享受 8 折优惠
c1.apply_discount(0.8)
c1.print_discount_info(0.8)
```
运行结果如下:
```
小明购买了饼干,价格为10元。
小明购买了小熊,价格为30元。
小明的预算不足,无法购买游戏机。
小红购买了娃娃,价格为50元。
小红购买了积木,价格为30元。
顾客姓名:小明
顾客年龄:20
顾客性别:男
购买商品清单:[('饼干', 10), ('小熊', 30)]
商品总价值:40元
顾客姓名:小红
顾客年龄:18
顾客性别:女
购买商品清单:[('娃娃', 50), ('积木', 30)]
商品总价值:80元
商品打折后总价值:32.0元
商品折扣率为0.8,应付金额为8.0元
```
阅读全文
相关推荐

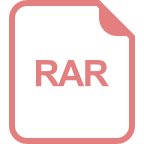
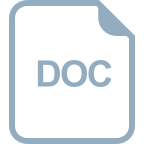
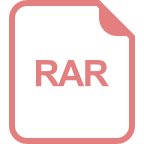
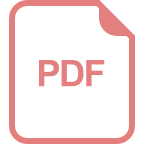
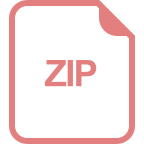
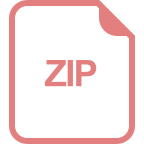
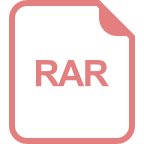
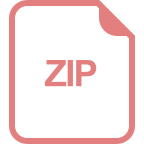
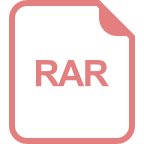
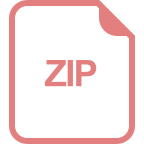
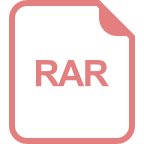