将一个pygame限时计分打地鼠游戏封装成函数
时间: 2023-06-13 09:05:48 浏览: 51
好的,以下是将一个 Pygame 限时计分打地鼠游戏封装成函数的示例代码:
```python
import pygame
import random
pygame.init()
pygame.font.init()
# 设置游戏窗口大小和标题
WIDTH = 800
HEIGHT = 600
WIN = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Whack-a-Mole")
# 设置游戏时钟
clock = pygame.time.Clock()
# 加载游戏音效和图片
hitsound = pygame.mixer.Sound("hit.wav")
background = pygame.image.load("background.jpg")
mole = pygame.image.load("mole.png")
# 设置字体
font = pygame.font.SysFont('Comic Sans MS', 30)
# 设置游戏变量
score = 0
time_left = 60
game_over = False
# 定义函数,生成地鼠
def generate_mole():
mole_x = random.randint(0, WIDTH - mole.get_width())
mole_y = random.randint(0, HEIGHT - mole.get_height())
return mole_x, mole_y
# 定义函数,显示得分和剩余时间
def display_score_time():
score_text = font.render("Score: " + str(score), True, (255, 255, 255))
time_text = font.render("Time Left: " + str(time_left), True, (255, 255, 255))
WIN.blit(score_text, (10, 10))
WIN.blit(time_text, (WIDTH - time_text.get_width() - 10, 10))
# 定义函数,显示游戏结束信息
def display_game_over():
game_over_text = font.render("Game Over! Your Final Score is " + str(score), True, (255, 255, 255))
WIN.blit(game_over_text, ((WIDTH - game_over_text.get_width()) / 2, (HEIGHT - game_over_text.get_height()) / 2))
# 游戏主循环
def whack_a_mole_game():
global score, time_left, game_over
mole_x, mole_y = generate_mole()
while not game_over:
clock.tick(60)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.MOUSEBUTTONDOWN:
mouse_x, mouse_y = pygame.mouse.get_pos()
if mole_x <= mouse_x <= mole_x + mole.get_width() and mole_y <= mouse_y <= mole_y + mole.get_height():
score += 1
hitsound.play()
mole_x, mole_y = generate_mole()
WIN.blit(background, (0, 0))
WIN.blit(mole, (mole_x, mole_y))
display_score_time()
if time_left == 0:
game_over = True
else:
time_left -= 1
pygame.display.update()
display_game_over()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
pygame.display.update()
# 运行游戏函数
whack_a_mole_game()
```
这个封装后的函数可以直接运行,会打开一个 Pygame 窗口,玩家需要在限定时间内尽可能地打地鼠,每打中一个地鼠就会得到一个分数。当时间到达上限后,游戏结束,窗口会显示玩家的最终得分。
相关推荐
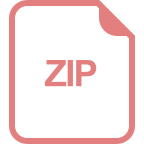












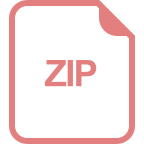
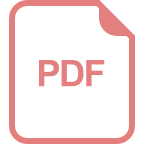