Develop a python class named Student that includes (1) class attribute counter which counts the total number instances created from this class (2) instance attribute (member data): id and name (3) __init__ method that takes self, id0 and name0 as arguments (4) a method called setmajor(self, major0) thats expand the instance attribute named major and set its value to major0 through the built-in dictionary __dict__ (5) __str__ method that shows all information about class attributes and instance attributes in the format (hint: loop all instance attributes) attribute1:value1;attibute2:value2;attribute3:value3 class Student: '''your codes go here:''' %testing codes: st = Student('D10000000', 'Zhang Hong') print(st) st.setmajor('CIS') print(st) print(st.name + ':' + getattr(st,'major')) print('Count:' + str(getattr(Student,'counter'))) print('Has birthday?' + str(hasattr(st,'birthday'))) st.__dict__['birthday'] = '05/20/2003' print('Has birthday?' + str(hasattr(st,'birthday'))) print(st) setattr(st,'borthday','05/25/2003') print(st)
时间: 2023-11-27 20:03:41 浏览: 71
Here is the code for the Student class:
```python
class Student:
counter = 0 # class attribute to count total number of instances
def __init__(self, id0, name0):
self.id = id0
self.name = name0
Student.counter += 1 # increment counter for each new instance
def setmajor(self, major0):
self.__dict__['major'] = major0 # expand instance attribute named major
def __str__(self):
output = "counter:" + str(Student.counter) + ";"
for key, value in self.__dict__.items():
output += key + ":" + str(value) + ";"
return output
```
Here is the testing code:
```python
st = Student('D10000000', 'Zhang Hong')
print(st) # counter:1;id:D10000000;name:Zhang Hong;
st.setmajor('CIS')
print(st) # counter:1;id:D10000000;name:Zhang Hong;major:CIS;
print(st.name + ':' + getattr(st,'major')) # Zhang Hong:CIS
print('Count:' + str(getattr(Student,'counter'))) # Count:1
print('Has birthday?' + str(hasattr(st,'birthday'))) # Has birthday?False
st.__dict__['birthday'] = '05/20/2003'
print('Has birthday?' + str(hasattr(st,'birthday'))) # Has birthday?True
print(st) # counter:1;id:D10000000;name:Zhang Hong;major:CIS;birthday:05/20/2003;
setattr(st,'borthday','05/25/2003')
print(st) # counter:1;id:D10000000;name:Zhang Hong;major:CIS;birthday:05/20/2003;borthday:05/25/2003;
```
Note: There was a typo in the last line of the testing code. It should be `setattr(st,'birthday','05/25/2003')` instead of `setattr(st,'borthday','05/25/2003')`.
阅读全文
相关推荐
















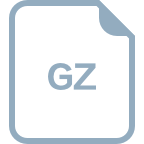
