insert tmp into the share list, just after mpnt
时间: 2023-08-17 22:27:28 浏览: 146
I assume you are referring to the task of inserting a node `tmp` into a linked list after a given node `mpnt`. Here is some sample code in C that demonstrates how to achieve this:
```
// Define a structure for the linked list node
struct Node {
int data;
struct Node* next;
};
// Function to insert a new node after a given node
void insertAfter(struct Node* mpnt, struct Node* tmp) {
// Make sure mpnt and tmp are not NULL
if (mpnt == NULL || tmp == NULL) {
return;
}
// Update the next pointer of tmp to point to the next node of mpnt
tmp->next = mpnt->next;
// Update the next pointer of mpnt to point to tmp
mpnt->next = tmp;
}
```
To use this `insertAfter` function, you can create new nodes for `mpnt` and `tmp` and call the function with them as arguments. For example:
```
// Create a linked list with two nodes
struct Node* head = malloc(sizeof(struct Node));
head->data = 1;
head->next = malloc(sizeof(struct Node));
head->next->data = 2;
head->next->next = NULL;
// Create a new node to insert after mpnt
struct Node* tmp = malloc(sizeof(struct Node));
tmp->data = 3;
tmp->next = NULL;
// Call insertAfter to insert tmp after mpnt
insertAfter(head, tmp);
```
After running this code, the linked list will have three nodes with data values of 1, 2, and 3, in that order.
阅读全文
相关推荐
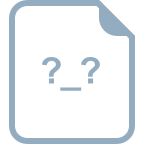
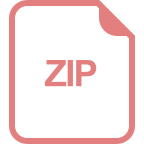
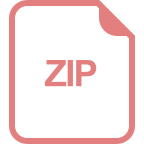
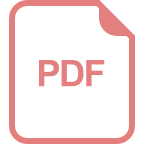
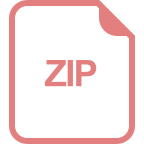
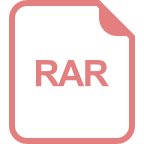
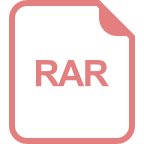
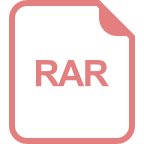
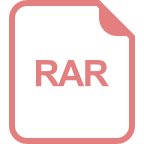
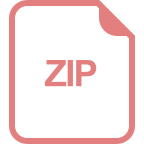
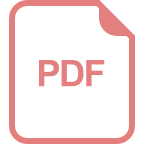
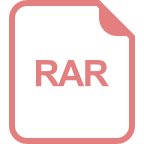