写一段pandas的基础代码
时间: 2023-09-16 15:15:04 浏览: 85
当然,我非常乐意为您提供 Pandas 基础代码。以下是一个示例代码块,可以帮助您开始使用 Pandas:
```
import pandas as pd
# 创建一个 Pandas 的数据帧
data = {'Name': ['Tom', 'Jack', 'Steve', 'Ricky'], 'Age': [28, 34, 29, 42]}
df = pd.DataFrame(data)
# 打印整个 DataFrame
print(df)
# 选取其中的一列
ages = df['Age']
print(ages)
# 选取其中的一行
row = df.iloc[1]
print(row)
```
这段代码展示了如何创建一个 Pandas 数据帧,选取数据帧中的一列和一行,并将它们打印出来。当然,Pandas 还有更多强大的功能和方法,供您进行数据处理和分析。
相关问题
python pandas简单代码
### Python Pandas 简单代码示例
#### 创建并操作 DataFrame
创建一个简单的 `DataFrame` 是使用 Pandas 进行数据分析的基础:
```python
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']}
df = pd.DataFrame(data)
print(df)
```
这段代码展示了如何通过字典来构建一个包含姓名、年龄和城市的数据框[^1]。
#### 数据类型的转换
有时需要改变列中的数据类型,这可以通过 `.astype()` 方法实现:
```python
df['Age'] = df['Age'].astype(str)
print(df.dtypes)
```
此段代码将 Age 列由整数型转为了字符串型[^2]。
#### 比较两个 DataFrame 中对应位置上的元素是否不同
如果想要比较两组数据之间的差异,则可以利用 `.ne()` 函数来进行逐项对比:
```python
other_data = {'Name': ['Alice', 'Bobby', 'Chris'],
'Age': [25, 31, 40],
'City': ['Boston', 'San Francisco', 'Seattle']}
other_df = pd.DataFrame(other_data)
comparison = df.ne(other_df)
print(comparison)
```
这里显示了怎样判断原始数据帧与其他数据帧之间各字段值的不同之处[^3]。
#### 将 DataFrame 导出到 Excel 文件
最后一步通常是把处理好的表格保存下来供后续查看或分享给他人。下面的例子说明了怎么设置日期格式并将结果存入 Excel 表格中:
```python
with pd.ExcelWriter('output.xlsx', date_format='YYYY-MM-DD') as writer:
df.to_excel(writer, sheet_name='Sheet1')
```
上述命令会按照指定的时间样式导出当前工作区内的数据至名为 output.xlsx 的电子表格文档里[^4]。
pandas数据可视化图表源代码
### Pandas 数据可视化 图表 示例 源代码
#### 折线图示例
为了展示如何利用 `pandas` 进行简单的折线图绘制,下面提供了一个基于时间序列数据的例子:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 创建时间序列数据
date_rng = pd.date_range(start='2023-10-01', end='2023-10-10', freq='D')
data = {'Value': [10, 15, 12, 18, 22, 28, 35, 40, 38, 42]}
df = pd.DataFrame(data, index=date_rng)
# 绘制折线图
plt.figure(figsize=(10, 6))
df.plot(kind='line', marker='o', color='blue')
plt.title('Time Series Data Visualization with Line Chart')
plt.xlabel('Date')
plt.ylabel('Values')
plt.grid(True)
plt.show()
```
此段代码展示了如何通过指定参数来调整图形样式并最终显示图表[^3]。
#### 散点图示例
对于散点图来说,则可以通过如下方式实现:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 构建随机数作为样本数据集
np.random.seed(0)
x = np.random.rand(50)
y = np.random.rand(50)
# 将其转换成 DataFrame 形式以便于操作
df_scatter = pd.DataFrame({'X': x, 'Y': y})
# 使用 plot 方法中的 kind 参数设置为 scatter 来生成散点图
df_scatter.plot(kind='scatter', x='X', y='Y', s=100 * df_scatter['Y'], alpha=0.5, c=['red'])
plt.title('Scatter Plot Example Using Pandas')
plt.xlabel('Random X Values')
plt.ylabel('Random Y Values')
plt.show()
```
这段代码说明了怎样创建一个带有不同大小和颜色标记的散点图[^1]。
#### 柱状图示例
最后给出一个关于柱状图的具体实例,这同样来自于之前提到的基础结构之上进行了扩展应用:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 定义基础数据框架
data_bar = {'name': ['John', 'Tom', 'Mary', 'Bob'],
'age': [25, 30, 20, 35],
'gender': ['M', 'M', 'F', 'M']}
df_bar = pd.DataFrame(data_bar)
# 调用 plot 函数,并指明 chart 类型为 bar (即柱状图),以及用于分类轴上的列名 name 和数值轴上的 age 列
ax = df_bar.plot(kind='bar', x='name', y='age', rot=0, figsize=(9, 7), colormap='viridis')
for p in ax.patches:
ax.annotate(str(p.get_height()), (p.get_x() * 1.005, p.get_height() * 1.005))
plt.title('Bar Chart Showing Ages of Individuals')
plt.xlabel('Names')
plt.ylabel('Ages')
plt.show()
```
上述代码不仅实现了基本的柱状图功能,还加入了额外的数据标签以增强可读性和美观度。
阅读全文
相关推荐



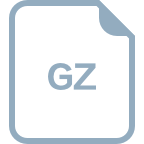








