请使用C语言实现队列的基本操作以及中文注释
时间: 2023-11-19 22:05:15 浏览: 30
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 定义队列结构体
typedef struct Queue {
int data[MAX_SIZE]; // 存储队列元素的数组
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue* queue) {
queue->front = queue->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue* queue) {
return queue->front == queue->rear;
}
// 判断队列是否已满
int isFull(Queue* queue) {
return (queue->rear + 1) % MAX_SIZE == queue->front;
}
// 入队
void enqueue(Queue* queue, int value) {
// 判断队列是否已满
if (isFull(queue)) {
printf("队列已满,无法入队。\n");
return;
}
// 将元素插入队尾
queue->data[queue->rear] = value;
// 队尾指针后移
queue->rear = (queue->rear + 1) % MAX_SIZE;
}
// 出队
int dequeue(Queue* queue) {
// 判断队列是否为空
if (isEmpty(queue)) {
printf("队列为空,无法出队。\n");
return -1;
}
// 获取队头元素
int value = queue->data[queue->front];
// 队头指针后移
queue->front = (queue->front + 1) % MAX_SIZE;
return value;
}
// 获取队头元素
int getFront(Queue* queue) {
// 判断队列是否为空
if (isEmpty(queue)) {
printf("队列为空,无法获取队头元素。\n");
return -1;
}
return queue->data[queue->front];
}
// 获取队尾元素
int getRear(Queue* queue) {
// 判断队列是否为空
if (isEmpty(queue)) {
printf("队列为空,无法获取队尾元素。\n");
return -1;
}
int rearIndex = (queue->rear - 1 + MAX_SIZE) % MAX_SIZE;
return queue->data[rearIndex];
}
int main() {
Queue queue;
initQueue(&queue);
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3);
printf("队头元素:%d\n", getFront(&queue));
printf("队尾元素:%d\n", getRear(&queue));
printf("出队元素:%d\n", dequeue(&queue));
printf("出队元素:%d\n", dequeue(&queue));
printf("出队元素:%d\n", dequeue(&queue));
printf("队列是否为空:%d\n", isEmpty(&queue));
return 0;
}
相关推荐
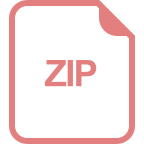














