linux进程间通信实例代码
时间: 2023-03-21 19:01:52 浏览: 136
以下是一个使用Linux进程间通信(IPC)机制的示例代码,包括使用管道(pipe)、共享内存(shared memory)和信号量(semaphore)进行进程间通信。
1. 使用管道(pipe)进行进程间通信:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#define BUFFER_SIZE 25
int main()
{
int fd[2];
pid_t pid;
char write_msg[BUFFER_SIZE] = "Greetings";
char read_msg[BUFFER_SIZE];
if (pipe(fd) == -1)
{
fprintf(stderr, "Pipe failed");
return 1;
}
pid = fork();
if (pid < 0)
{
fprintf(stderr, "Fork failed");
return 1;
}
if (pid > 0) // Parent process
{
close(fd[0]);
write(fd[1], write_msg, BUFFER_SIZE);
close(fd[1]);
}
else // Child process
{
close(fd[1]);
read(fd[0], read_msg, BUFFER_SIZE);
printf("Received message: %s\n", read_msg);
close(fd[0]);
}
return 0;
}
```
2. 使用共享内存(shared memory)进行进程间通信:
```
#include <stdio.h>
#include <stdlib.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main()
{
int shmid;
key_t key = 1234;
char *shm, *s;
if ((shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666)) < 0)
{
perror("shmget");
exit(1);
}
if ((shm = shmat(shmid, NULL, 0)) == (char *) -1)
{
perror("shmat");
exit(1);
}
printf("Enter message: ");
for (s = shm; (*s = getchar()) != '\n'; s++)
;
*s = '\0';
printf("You entered: %s\n", shm);
shmdt(shm);
return 0;
}
```
3. 使用信号量(semaphore)进行进程间通信:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#define MAX_RETRIES 10
union semun {
int val;
struct semid_ds *buf;
unsigned short *array;
};
int main()
{
key_t key = 1234;
int semid, semval;
union semun arg;
struct sembuf sembuf;
if ((semid = semget(key, 1, IPC_CREAT | 0666)) == -1)
{
perror("semget");
exit(1);
}
arg.val = 1;
if (semctl(semid, 0, SETVAL, arg) == -1)
{
perror("semctl");
exit(1);
}
if ((semval = semctl(semid, 0, GETVAL, 0)) == -1)
{
perror("semctl");
exit(1);
}
printf("Semaphore value before decrement: %d\n", semval);
sembuf.sem_num = 0;
sembuf.sem_op = -1;
sembuf.sem_flg =
相关推荐
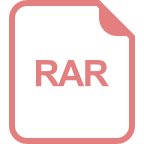
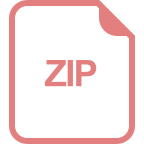
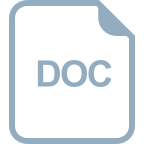
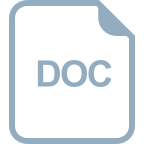
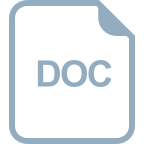
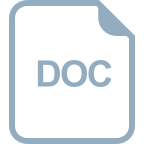
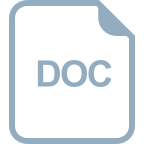
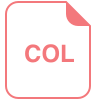
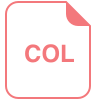
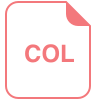
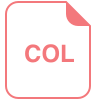
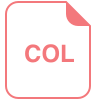




