stm32用c语言控制直流电机,按键1加速 按键2减速 按键3控制方向
时间: 2023-11-19 16:19:28 浏览: 81
好的,这个问题我可以回答。首先,需要连接一个电机驱动器来控制直流电机。然后,通过STM32的GPIO模块来读取按键状态,根据按键状态来控制电机的运动状态。
以下是一个大致的代码示例:
```c
#include "stm32f10x.h"
#define MOTOR_FORWARD 1
#define MOTOR_BACKWARD 2
int motor_direction = MOTOR_FORWARD;
int motor_speed = 0;
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
/* 配置PA0-PA2为输入模式 */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void TIM_Configuration(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
/* 配置定时器2为计数模式 */
TIM_TimeBaseStructure.TIM_Period = 65535;
TIM_TimeBaseStructure.TIM_Prescaler = 72 - 1;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
/* 开启定时器2 */
TIM_Cmd(TIM2, ENABLE);
}
void set_motor_direction(int direction)
{
if (direction == MOTOR_FORWARD)
{
/* 设置正转方向 */
// TODO: 设置电机驱动器控制信号
motor_direction = MOTOR_FORWARD;
}
else if (direction == MOTOR_BACKWARD)
{
/* 设置反转方向 */
// TODO: 设置电机驱动器控制信号
motor_direction = MOTOR_BACKWARD;
}
}
void set_motor_speed(int speed)
{
/* 设置电机转速 */
// TODO: 设置电机驱动器控制信号
motor_speed = speed;
}
int main(void)
{
GPIO_Configuration();
TIM_Configuration();
while (1)
{
/* 检测按键状态 */
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET)
{
/* 按键1按下,加速 */
set_motor_speed(motor_speed + 10);
}
else if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_1) == RESET)
{
/* 按键2按下,减速 */
set_motor_speed(motor_speed - 10);
}
else if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_2) == RESET)
{
/* 按键3按下,改变方向 */
if (motor_direction == MOTOR_FORWARD)
{
set_motor_direction(MOTOR_BACKWARD);
}
else
{
set_motor_direction(MOTOR_FORWARD);
}
}
/* 延时一段时间 */
for (int i = 0; i < 1000000; i++);
}
}
```
需要根据具体的电机驱动器和控制信号来修改TODO部分的代码。另外,需要注意电机的启动和停止,需要在set_motor_speed函数中添加对应的代码。
阅读全文
相关推荐
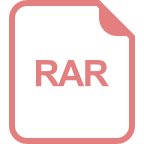
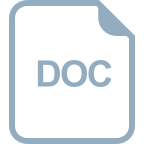
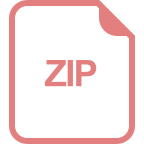
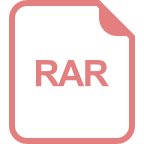
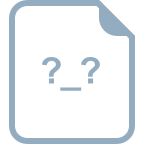
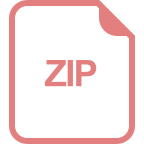
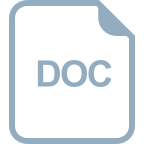
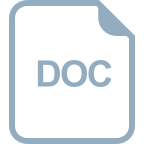
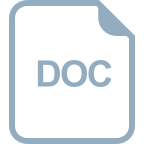
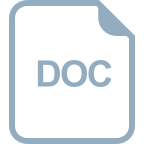
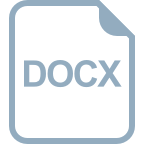
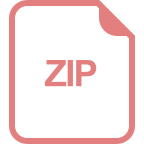