preparedstatement.setobject
时间: 2023-04-26 12:02:51 浏览: 121
`PreparedStatement.setObject` 是一个Java中的方法,用于设置预编译SQL语句中的占位符的值。它可以接受任何类型的参数并将其转换为合适的数据库类型,因此可以用于设置各种不同类型的参数值。例如,您可以使用此方法将整数、字符串、日期等数据类型设置为SQL查询语句中的参数。
相关问题
帮我优化这段代码,谢谢package com.neutech.utils; import java.sql.*; import java.util.ArrayList; import java.util.List; public class Jdbcutil { private static final String DRIVERNAME = "org.apache.hive.jdbc.HiveDriver"; private static final String URL = "jdbc:hive2://192.168.44.199:10000/test"; private static final String USERNAME = "root"; private static final String PASSWORD = "200812"; public static Connection getConnection() throws ClassNotFoundException { Class.forName(DRIVERNAME); Connection connection = null; try { connection = DriverManager.getConnection(URL,USERNAME,PASSWORD); } catch (SQLException throwables) { throwables.printStackTrace(); } return connection; } public static <T> List<T> execuetQuery(String sql, RowMap<T> rowMap, Object... objs) throws ClassNotFoundException, SQLException { // 建立连接 Connection connection = getConnection(); PreparedStatement preparedStatement = connection.prepareStatement(sql); // 注入额外条件 if(objs != null){ for(int i = 0;i <= objs.length;i++){ preparedStatement.setObject(i + 1,objs[i]); } } ResultSet resultSet = preparedStatement.executeQuery(); ArrayList<T> list = new ArrayList<>(); while(resultSet.next()){ T t = rowMap.RowMapping(resultSet); list.add(t); } return list; } public static void close(Connection connection,PreparedStatement preparedStatement,ResultSet resultSet) throws SQLException { connection.close(); preparedStatement.close(); resultSet.close(); } }
这段代码看起来已经很简洁了,但是还是可以做一些小优化:
1. 将常量放到配置文件中,方便修改和维护,比如可以使用 Properties 或者 YAML 等配置方式。
2. 在连接数据库时,可以使用连接池技术,避免频繁地创建和销毁连接,提高性能。
3. 在执行 SQL 语句时,可以使用 PreparedStatement 的批处理功能,将多个 SQL 语句一次性发送到数据库,减少网络传输开销。
4. 在处理异常时,可以考虑使用日志框架,比如 Log4j 或者 SLF4J 等,将异常信息记录到日志中,方便排查问题。
5. 在关闭资源时,可以使用 try-with-resources 语句,避免手动关闭资源时出现异常导致资源没有正确关闭的问题。
以下是修改后的代码:
```
package com.neutech.utils;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
public class JdbcUtil {
private static final String CONFIG_FILE = "jdbc.properties";
private static Properties properties = new Properties();
private static String driverName;
private static String url;
private static String username;
private static String password;
static {
try {
properties.load(JdbcUtil.class.getClassLoader().getResourceAsStream(CONFIG_FILE));
driverName = properties.getProperty("jdbc.driverName");
url = properties.getProperty("jdbc.url");
username = properties.getProperty("jdbc.username");
password = properties.getProperty("jdbc.password");
Class.forName(driverName);
} catch (Exception e) {
throw new RuntimeException("Failed to initialize JdbcUtil.", e);
}
}
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(url, username, password);
}
public static <T> List<T> executeQuery(String sql, RowMap<T> rowMap, Object... objs) throws SQLException {
try (Connection connection = getConnection();
PreparedStatement preparedStatement = connection.prepareStatement(sql)) {
if (objs != null) {
for (int i = 0; i < objs.length; i++) {
preparedStatement.setObject(i + 1, objs[i]);
}
}
try (ResultSet resultSet = preparedStatement.executeQuery()) {
List<T> list = new ArrayList<>();
while (resultSet.next()) {
T t = rowMap.RowMapping(resultSet);
list.add(t);
}
return list;
}
}
}
public static void close(Connection connection, PreparedStatement preparedStatement, ResultSet resultSet) {
try {
if (resultSet != null) {
resultSet.close();
}
} catch (SQLException e) {
// ignore
}
try {
if (preparedStatement != null) {
preparedStatement.close();
}
} catch (SQLException e) {
// ignore
}
try {
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
// ignore
}
}
}
```
以上修改参考了 Java 开发手册和阿里巴巴 Java 开发手册的相关规范。
preparedstatement的setobject
setObject是PreparedStatement对象的方法之一,用于设置参数的值。它可以设置任何类型的参数值,包括字符串、日期、BigDecimal等等,它的使用方式类似于setString、setInt等其他设置参数的方法,但是它使用更为灵活。
相关推荐
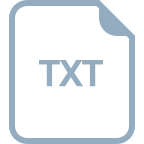
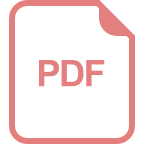












