将大写字母排序python_Python中sorted()排序与字母大小写的问题
时间: 2023-12-06 11:44:52 浏览: 138
在Python中,可以使用sorted()函数来对字符串中的大写字母进行排序。下面是示例代码:
```python
s = "HelloWorld"
upper_chars = sorted(filter(str.isupper, s))
print(upper_chars)
```
输出结果为:
```
['H', 'W']
```
其中,filter(str.isupper, s)函数用来过滤出字符串s中的大写字母,然后使用sorted()函数对其进行排序。
如果要忽略字母的大小写,可以在比较时将所有字母都转换为小写或大写,然后再进行比较。例如:
```python
s = "HelloWorld"
upper_chars = sorted(filter(str.isupper, s), key=lambda x: x.lower())
print(upper_chars)
```
输出结果为:
```
['H', 'W']
```
其中,key=lambda x: x.lower()函数指定了排序时的比较方式,将字母都转换为小写字母进行比较。
相关问题
Python编写一个程序,随机生成1000个字母,包含大写字母和小写字母,然后统计各个字母出现的次数,统计时忽略字母的大小写,最后将统计结果按照字母出现的次数从高到低排序输出。
在Python中,你可以使用`collections.Counter`类来轻松完成这个任务。这是一个字典子类,用于计数可哈希对象。以下是实现此功能的一个示例程序:
```python
import string
import random
from collections import Counter
# 随机生成包含大写字母、小写字母的字符串
random_string = ''.join(random.choices(string.ascii_letters, k=1000))
# 使用Counter统计字母出现次数,并忽略大小写
letter_counts = Counter(random_string.lower())
# 按照字母出现次数降序排列并打印结果
sorted_counts = sorted(letter_counts.items(), key=lambda x: x[1], reverse=True)
for letter, count in sorted_counts:
print(f"{letter}: {count}")
```
运行这个程序后,它会输出每个字母及其出现的次数,从最常出现的字母开始。
python:输入一个任意字符串。其中包含有大小写字母,编写程序将小写字母放在最前面,大写字母放在最后面。
你可以使用Python的内置函数`sorted()`以及列表推导式来实现这个功能。首先,创建一个辅助函数来判断字符是否为大写字母:
```python
def is_uppercase(char):
return char.isupper()
```
然后,定义主函数,接受字符串作为输入,对字符串中的字符按字母顺序排序,并保持区分大小写的特性:
```python
def rearrange_string(input_str):
# 使用列表推导式,先筛选出所有小写字母,再添加大写字母
sorted_chars = [char for char in input_str if not is_uppercase(char)] + [char for char in input_str if is_uppercase(char)]
return ''.join(sorted_chars)
```
现在你可以调用`rearrange_string()`函数并传入你需要处理的字符串:
```python
input_string = input("请输入一个包含大小写字母的字符串: ")
result = rearrange_string(input_string)
print(f"处理后的字符串: {result}")
阅读全文
相关推荐
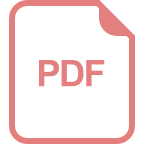
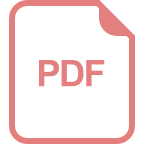
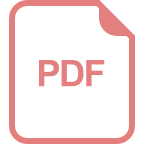










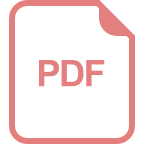