C语言 用结构体从键盘中输入3个学生的学号,姓名和3门课的成绩,输出每个学生的姓名和三门课成绩的平均值
时间: 2024-01-02 19:05:00 浏览: 125
以下是一个解决方案:
```c
#include <stdio.h>
// 定义学生结构体
struct Student {
int id;
char name[20];
float score[3];
float average;
};
int main() {
struct Student student[3];
// 从键盘中输入学生信息
for (int i = 0; i < 3; i++) {
printf("请输入第%d个学生的学号、姓名和三门课的成绩:\n", i+1);
scanf("%d %s %f %f %f", &student[i].id, student[i].name, &student[i].score[0], &student[i].score[1], &student[i].score[2]);
// 计算平均值
student[i].average = (student[i].score[0] + student[i].score[1] + student[i].score[2]) / 3.0;
}
// 输出每个学生的姓名和成绩
printf("学号\t姓名\t成绩1\t成绩2\t成绩3\t平均成绩\n");
for (int i = 0; i < 3; i++) {
printf("%d\t%s\t%.1f\t%.1f\t%.1f\t%.1f\n", student[i].id, student[i].name, student[i].score[0], student[i].score[1], student[i].score[2], student[i].average);
}
return 0;
}
```
这个程序使用结构体来表示学生信息。在循环中,使用 `scanf` 从键盘中输入学生信息,并计算平均值。最后,使用 `printf` 输出每个学生的学号、姓名、三门课成绩和平均值。注意,为了方便对齐,我们使用了 `\t` 水平制表符来分隔输出。
阅读全文
相关推荐
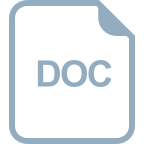
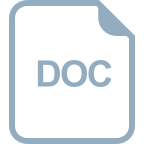
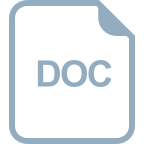















