android 蓝牙一对多连接实现源码
时间: 2023-07-26 09:03:23 浏览: 62
以下是一个简单的 Android 蓝牙一对多连接示例:
1. MainActivity.java
```
public class MainActivity extends AppCompatActivity {
private BluetoothAdapter mBluetoothAdapter;
private ArrayList<BluetoothSocket> mSockets = new ArrayList<>();
private ArrayList<BluetoothDevice> mDevices = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 获取蓝牙适配器
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
// 扫描蓝牙设备
scanDevices();
}
// 扫描蓝牙设备
private void scanDevices() {
mBluetoothAdapter.startDiscovery();
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
registerReceiver(mReceiver, filter);
}
// 广播接收器,用于获取扫描到的蓝牙设备
private final BroadcastReceiver mReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
if (!mDevices.contains(device)) {
mDevices.add(device);
connectDevice(device);
}
}
}
};
// 连接蓝牙设备
private void connectDevice(BluetoothDevice device) {
new Thread(() -> {
try {
BluetoothSocket socket = device.createRfcommSocketToServiceRecord(UUID.randomUUID());
socket.connect();
mSockets.add(socket);
} catch (IOException e) {
e.printStackTrace();
}
}).start();
}
// 发送消息给所有连接的设备
private void sendMessages(String message) {
for (BluetoothSocket socket : mSockets) {
try {
OutputStream outputStream = socket.getOutputStream();
outputStream.write(message.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Override
protected void onDestroy() {
super.onDestroy();
unregisterReceiver(mReceiver);
for (BluetoothSocket socket : mSockets) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
2. activity_main.xml
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/btn_send"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="发送消息"/>
</LinearLayout>
```
在上面的示例中,我们使用 `BluetoothAdapter` 获取蓝牙适配器,并调用 `startDiscovery()` 方法开始扫描蓝牙设备。然后,我们使用 `BroadcastReceiver` 监听 `ACTION_FOUND` 广播,获取扫描到的蓝牙设备,并使用 `connectDevice()` 方法连接设备。
连接设备时,我们使用 `UUID.randomUUID()` 方法生成一个 UUID,并以此创建一个 `BluetoothSocket`。然后,我们调用 `connect()` 方法连接设备,并将连接的 `BluetoothSocket` 添加到 `mSockets` 列表中。
最后,我们使用 `sendMessages()` 方法发送消息给所有连接的设备。在 `onDestroy()` 方法中,我们注销 `BroadcastReceiver` 并关闭所有连接的 `BluetoothSocket`。
相关推荐
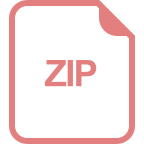
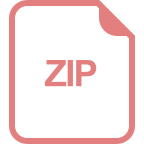














