Input: xyz: input points position data, [B, C, N] points: input points data, [B, D, N] Return: new_xyz: sampled points position data, [B, C, S] new_points_concat: sample points feature data, [B, D', S]解释
时间: 2024-01-20 18:04:40 浏览: 65
这是一个函数的输入和输出说明,函数的作用是对输入的点云进行采样,输出采样后的点云位置和特征。
具体解释如下:
输入:
- xyz: 输入点云的位置数据,形状为 [B, C, N],其中B是batch size,C是坐标维度(通常为3,表示x、y、z三个维度),N是原始点云中点的数量。
- points: 输入点云的特征数据,形状为 [B, D, N],其中D是特征维度,N是原始点云中点的数量。
输出:
- new_xyz: 采样后的点云位置数据,形状为 [B, C, S],其中S是采样后点的数量。
- new_points_concat: 采样后的点云特征数据,形状为 [B, D', S],其中D'是新的特征维度,S是采样后点的数量。在该函数中,通常会对每个采样点的邻域进行特征聚合,因此D'通常会大于D。
总之,该函数的作用是对输入的点云进行降采样,将点云的密度和特征维度降低,以便于后续处理。
相关问题
Input: xyz1: input points position data, [B, C, N] xyz2: sampled input points position data, [B, C, S] points1: input points data, [B, D, N] points2: input points data, [B, D, S] Return: new_points: upsampled points data, [B, D', N] # 上采样后的点解释
这是一个函数的输入和输出说明,函数的作用是对输入的采样点云进行上采样,将采样点云的特征插值到原始点云上。
具体解释如下:
输入:
- xyz1: 输入点云的位置数据,形状为 [B, C, N],其中B是batch size,C是坐标维度(通常为3,表示x、y、z三个维度),N是原始点云中点的数量。
- xyz2: 输入采样点云的位置数据,形状为 [B, C, S],其中S是采样点的数量。
- points1: 输入点云的特征数据,形状为 [B, D, N],其中D是特征维度,N是原始点云中点的数量。
- points2: 输入采样点云的特征数据,形状为 [B, D, S],其中D是特征维度,S是采样点的数量。
输出:
- new_points: 上采样后的点云特征数据,形状为 [B, D', N],其中D'是新的特征维度,N是原始点云中点的数量。在该函数中,通常会对每个原始点的邻域进行插值操作,将采样点云的特征插值到原始点上,以得到更高密度的点云。
总之,该函数的作用是对输入的采样点云进行上采样,将采样点云的特征插值到原始点云上,以得到更高密度的点云。
import os import sys import numpy as np def creat_pcd(input_path, output_path): #Lodaing txt Full_Data = np.loadtxt(input_path) #Creating pcd if os.path.exists(output_path): os.remove(output_path) Output_Data = open(output_path, 'a') Output_Data.write('# .PCD v0.7 - Point Cloud Data file format\nVERSION 0.7\nFIELDS x y z rgba\nSIZE 4 4 4 4\nTYPE F F F U\nCOUNT 1 1 1 1') string = '\nWIDTH ' + str(Full_Data.shape[0]) Output_Data.write(string) Output_Data.write('\nHEIGHT 1\nVIEWPOINT 0 0 0 1 0 0 0') string = '\nPOINTS ' + str(Full_Data.shape[0]) Output_Data.write(string) Output_Data.write('\nDATA ascii') for j in range(Full_Data.shape[0]): R=Full_Data[j,1] G=Full_Data[j,1] B=Full_Data[j,2] value = (int(R) << 16 | int(G) << 8 | int(B)) string = ('\n' + str(Full_Data[j,0]) + ' ' + str(Full_Data[j, 1]) + ' ' +str(Full_Data[j, 2]) + ' ' + str(value)) Output_Data.write(string) Output_Data.close() print('--------------Completed--------------') a = input("请输入TXT文件路径:")#文件路径中斜杆使用"/",比如:D:/pcl/points.txt b = input("请输入PCD文件保存路径:")#比如:D:/pcl/points.pcd creat_pcd(a, b)对这些代码优化
以下是对代码的优化建议:
1. 引入模块应当分行,不要写在同一行。
2. 应使用 with 语句打开文件,可以自动关闭文件,避免忘记关闭文件而导致资源泄露。
3. 在写入文件时应当使用字符串格式化来避免繁琐的字符串拼接操作,提高代码可读性。
4. 变量名应该具有具体的描述性,便于代码阅读和理解。
5. 字段名称和数据类型定义可以提取为常量或变量,便于修改和维护。
6. 可以使用 argparse 模块来处理命令行参数,更加方便用户使用。
下面是优化后的代码:
```python
import os
import sys
import argparse
import numpy as np
PCD_FIELDS = ['x', 'y', 'z', 'rgba']
PCD_SIZE = [4, 4, 4, 4]
PCD_TYPE = ['F', 'F', 'F', 'U']
PCD_COUNT = [1, 1, 1, 1]
PCD_VERSION = '0.7'
def create_pcd(input_path, output_path):
# Loading txt
full_data = np.loadtxt(input_path)
# Creating pcd
if os.path.exists(output_path):
os.remove(output_path)
with open(output_path, 'a') as output_data:
output_data.write(f"# .PCD v{PCD_VERSION} - Point Cloud Data file format\n")
output_data.write("VERSION {}\n".format(PCD_VERSION))
output_data.write("FIELDS {}\n".format(" ".join(PCD_FIELDS)))
output_data.write("SIZE {}\n".format(" ".join(map(str, PCD_SIZE))))
output_data.write("TYPE {}\n".format(" ".join(PCD_TYPE)))
output_data.write("COUNT {}\n".format(" ".join(map(str, PCD_COUNT))))
output_data.write("WIDTH {}\n".format(full_data.shape[0]))
output_data.write("HEIGHT 1\n")
output_data.write("VIEWPOINT 0 0 0 1 0 0 0\n")
output_data.write("POINTS {}\n".format(full_data.shape[0]))
output_data.write("DATA ascii\n")
for j in range(full_data.shape[0]):
r = full_data[j, 1]
g = full_data[j, 1]
b = full_data[j, 2]
value = (int(r) << 16 | int(g) << 8 | int(b))
output_data.write("{:.6f} {:.6f} {:.6f} {:d}\n".format(full_data[j, 0], full_data[j, 1], full_data[j, 2], value))
print('--------------Completed--------------')
def parse_args():
parser = argparse.ArgumentParser(description='Convert txt file to pcd file.')
parser.add_argument('input_path', type=str, help='input txt file path')
parser.add_argument('output_path', type=str, help='output pcd file path')
return parser.parse_args()
if __name__ == '__main__':
args = parse_args()
create_pcd(args.input_path, args.output_path)
```
使用 argparse 模块可以从命令行方便地输入文件路径,例如:
```bash
python create_pcd.py D:/pcl/points.txt D:/pcl/points.pcd
```
阅读全文
相关推荐
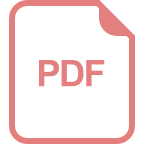
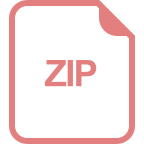
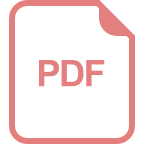
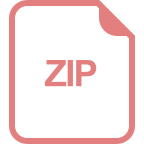


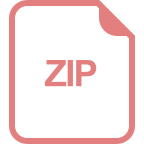
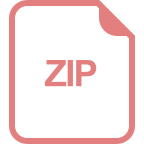
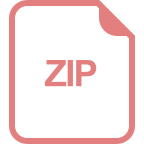
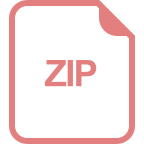
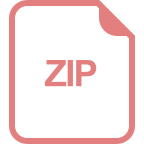
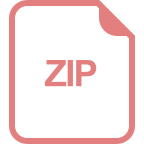
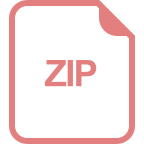
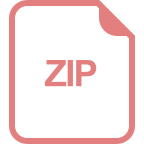
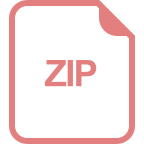
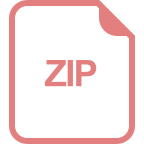
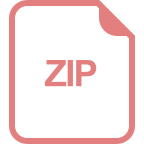