用python生成一段代码读取txt文件(文件包括学号,姓名,成绩),统计出每个人成绩最高的三次分数,代码用模块函数实现
时间: 2023-12-10 13:39:38 浏览: 89
可以使用Python内置的csv模块来读取包含学号、姓名和成绩的txt文件,然后使用字典来存储每个学生的成绩,最后找到每个学生的最高三次分数。以下是实现代码:
```python
import csv
def read_file(file_name):
# 读取txt文件
with open(file_name, "r") as csvfile:
reader = csv.reader(csvfile)
# 跳过文件的第一行(表头)
next(reader)
# 创建一个字典用来存储每个学生的成绩
scores = {}
for row in reader:
stu_id, stu_name, score = row
# 如果当前学生不存在于字典中,则添加该学生
if stu_id not in scores:
scores[stu_id] = {"name": stu_name, "scores": []}
# 将该学生的成绩添加到字典中
scores[stu_id]["scores"].append(int(score))
return scores
def get_top_scores(scores):
top_scores = {}
# 遍历每个学生的成绩
for stu_id in scores:
# 获取该学生的所有成绩并排序
stu_scores = sorted(scores[stu_id]["scores"], reverse=True)
# 取出该学生的最高三次分数
top_scores[stu_id] = stu_scores[:3]
return top_scores
if __name__ == "__main__":
# 测试代码
scores = read_file("scores.txt")
top_scores = get_top_scores(scores)
for stu_id in top_scores:
print("学号:{},姓名:{},最高三次分数:{}".format(stu_id, scores[stu_id]["name"], top_scores[stu_id]))
```
上述代码中,`read_file()`函数用来读取txt文件并将每个学生的成绩存储到字典中,`get_top_scores()`函数用来找到每个学生的最高三次分数,最后在主程序中调用这两个函数并打印结果。
阅读全文
相关推荐
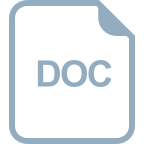
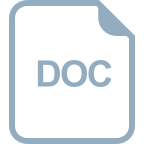
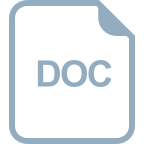




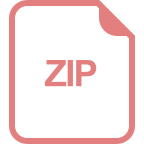
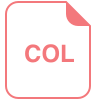
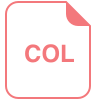
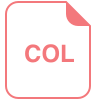
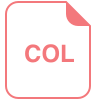
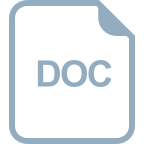
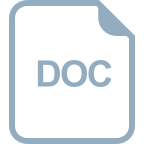
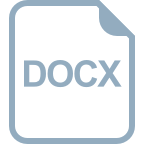
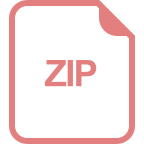