python if 字符串 or 字符串
时间: 2023-10-08 14:04:01 浏览: 77
在 Python 中,if 语句可以用来检查一个条件是否为 True。当条件为 True 时,if 语句中的代码块会被执行。
要检查多个条件,可以使用逻辑运算符,如 and 和 or。如果你想要检查两个字符串是否都为 True,可以使用 and 运算符,如下所示:
```python
if string1 and string2:
# do something
```
如果你想要检查两个字符串中至少一个为 True,可以使用 or 运算符,如下所示:
```python
if string1 or string2:
# do something
```
注意,在这里的字符串是被视为 True 的,只要不是空字符串或者 None,就被视为 True。
相关问题
python 寻找字符串里是否有指定字符串
可以使用 Python 的 in 关键字来判断一个字符串是否包含另一个字符串。例如:
```python
string1 = "hello world"
string2 = "world"
if string2 in string1:
print("string2 is found in string1")
else:
print("string2 is not found in string1")
```
输出结果为:
```
string2 is found in string1
```
如果要判断字符串里是否有多个指定字符串,可以使用多个 in 关键字,例如:
```python
string1 = "hello world"
string2 = "world"
string3 = "python"
if string2 in string1 and string3 in string1:
print("string2 and string3 are both found in string1")
else:
print("string2 and/or string3 are not found in string1")
```
输出结果为:
```
string2 and/or string3 are not found in string1
```
python回文字符串
回文字符串是指从左往右和从右往左读都一样的字符串。在Python中,可以使用reversed()函数和字符串拼接来判断一个字符串是否是回文字符串。下面是一个示例代码:
```python
def is_palindrome(string):
return string == ''.join(list(reversed(string)))
```
此外,如果你想找出一个字符串中的最长回文子串,可以使用以下方法。首先,将原串逆转并拼接到原串中,并以特殊字符(例如'#')分隔原串和逆转串,以避免内部字符串干扰。然后,使用最长公共前缀算法(Longest Common Prefix,简称LCP)来找到最长回文字符串的长度。下面是一个示例代码:
```python
def longest_palindrome_prefix(s):
if not s:
return 0
s = s + '#' + s[::-1] + '$'
i = 0
j = -1
nxt = [0] * len(s)
nxt[0] = -1
while i < len(s) - 1:
if j == -1 or s[i] == s[j]:
i += 1
j += 1
nxt[i] = j
else:
j = nxt[j]
return nxt[len(s) - 1]
```
阅读全文
相关推荐
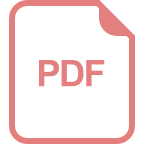
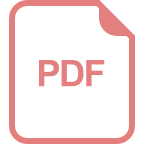
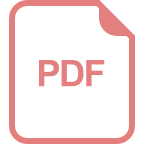
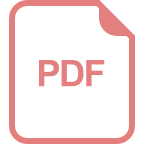
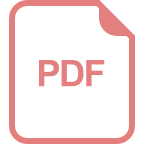
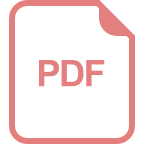
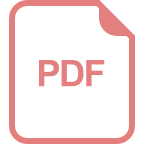
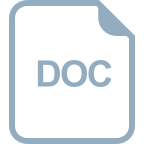





