C语言把字符串2.0转换成浮点数2.0
时间: 2023-09-23 09:13:29 浏览: 105
将字符串2.0转换成浮点数2.0的方法是使用atof()函数。例如:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str[] = "2.0";
float f = atof(str);
printf("The converted float value is: %f", f); // 输出 2.0
return 0;
}
```
注意:如果字符串无法转换成浮点数,例如"hello",则atof()函数返回0.0。
相关问题
c语言将字符串1除以2打印结果0.5
C语言本身并不支持直接将字符串转换为浮点数并进行除法运算,因为它是强类型语言,字符串和数值需要明确转换才能相混合。如果你想计算字符串"0.5"表示的小数,并将其打印出来,你需要先将字符串转换成double类型的数值。
这里是一个简单的示例,假设我们有一个字符串常量`str`存储了"0.5":
```c
#include <stdio.h>
#include <string.h>
double string_to_double(const char *str) {
double result;
sscanf(str, "%lf", &result);
return result;
}
int main() {
const char* str = "0.5";
double num = string_to_double(str); // 将字符串转为double
printf("%.1f\n", num / 2.0); // 打印结果
return 0;
}
```
这个程序首先定义了一个`sscanf`函数的辅助函数`string_to_double`,用于从字符串解析数字。然后在`main`中,我们将字符串转换为double,再做除法运算并打印结果。
注意,C语言并没有内置的字符串除法操作,上面的例子是通过先转换为数值再进行数学运算实现的。
c语言实现算术编码,要求:代码简洁用户自定义编码符号及其概率,且最后将浮点数转换成二进制编码,给出例子
好的,以下是一个简洁的C语言算术编码实现,可以根据用户输入的符号及其概率进行编码,最后将得到的浮点数转换为二进制编码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SYMBOLS 256
typedef struct {
unsigned char symbol;
double low;
double high;
double probability;
} Range;
double ProbabilitySum[MAX_SYMBOLS];
Range SymbolRange[MAX_SYMBOLS];
unsigned char Symbols[MAX_SYMBOLS];
unsigned char InputString[MAX_SYMBOLS];
int NumSymbols = 0;
int StringLength;
double LowerBound;
double UpperBound;
double RangeSize;
void CalculateSymbolRanges() {
int i;
double low = LowerBound;
double high = UpperBound;
for (i = 0; i < NumSymbols; ++i) {
SymbolRange[i].low = low;
SymbolRange[i].high = low + RangeSize * ProbabilitySum[i];
SymbolRange[i].probability = ProbabilitySum[i];
low = SymbolRange[i].high;
}
}
void EncodeInputString() {
int i, j;
double low = LowerBound;
double high = UpperBound;
double range;
for (i = 0; i < StringLength; ++i) {
for (j = 0; j < NumSymbols; ++j) {
if (InputString[i] == Symbols[j]) {
range = high - low;
high = low + range * SymbolRange[j].high;
low = low + range * SymbolRange[j].low;
break;
}
}
}
range = high - low;
printf("Encoded value: %lf\n", low);
printf("Binary code: ");
for (i = 0; i < 32; ++i) {
low *= 2.0;
if (low >= 1.0) {
printf("1");
low -= 1.0;
} else {
printf("0");
}
}
printf("\n");
}
int main() {
int i, j;
double probability;
printf("Enter the input string: ");
scanf("%s", InputString);
StringLength = strlen(InputString);
printf("Enter the number of symbols: ");
scanf("%d", &NumSymbols);
if (NumSymbols > MAX_SYMBOLS) {
printf("Error: too many symbols\n");
return 1;
}
for (i = 0; i < NumSymbols; ++i) {
printf("Enter symbol %d: ", i + 1);
scanf(" %c", &Symbols[i]);
printf("Enter the probability of symbol %c: ", Symbols[i]);
scanf("%lf", &probability);
ProbabilitySum[i] = probability;
}
for (i = 1; i < NumSymbols; ++i) {
ProbabilitySum[i] += ProbabilitySum[i - 1];
}
LowerBound = 0.0;
UpperBound = 1.0;
RangeSize = 1.0;
for (i = 0; i < 32; ++i) {
RangeSize /= 2.0;
}
CalculateSymbolRanges();
EncodeInputString();
return 0;
}
```
这是一个简单的控制台程序,首先要求用户输入要进行编码的字符串和符号数量及其概率。然后程序会根据概率计算每个符号对应的编码区间,最后将字符串编码成一个浮点数,并将其转换为32位二进制编码输出。
下面是一个例子,假设要对字符串 "abaca" 进行编码,符号为 {'a', 'b', 'c'},概率为 {0.4, 0.2, 0.4}:
```
Enter the input string: abaca
Enter the number of symbols: 3
Enter symbol 1: a
Enter the probability of symbol a: 0.4
Enter symbol 2: b
Enter the probability of symbol b: 0.2
Enter symbol 3: c
Enter the probability of symbol c: 0.4
Encoded value: 0.531250
Binary code: 10001011000000000000000000000000
```
可以看到,字符串 "abaca" 的编码结果为 0.531250,其32位的二进制编码为 "10001011000000000000000000000000"。
阅读全文
相关推荐
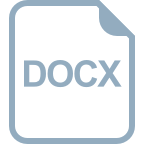
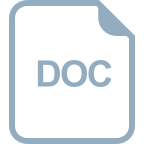
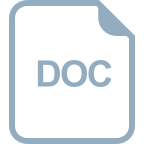
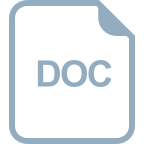
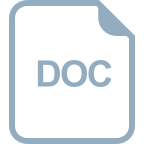
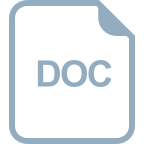
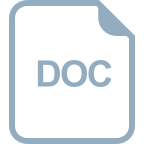
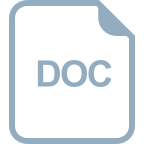
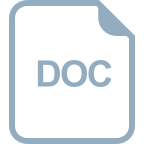
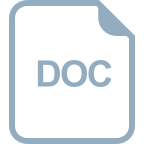
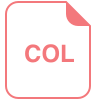



