自定义分页查询的Mapper
时间: 2023-12-07 20:02:34 浏览: 99
在MyBatis中,自定义分页查询的Mapper可以通过以下步骤实现:
1. 在Mapper.xml文件中定义自定义分页查询的SQL语句,例如:
```
<select id="findUsers" resultType="com.example.User">
SELECT * FROM users
LIMIT #{start}, #{pageSize}
</select>
```
其中,#{start}和#{pageSize}是动态参数,分别表示查询起始位置和每页记录数。
2. 在Mapper接口中定义对应方法,例如:
```
public interface UserMapper {
List<User> findUsers(@Param("start") int start, @Param("pageSize") int pageSize);
}
```
其中,@Param注解用于将方法参数与SQL语句中的动态参数对应。
3. 编写分页查询的工具类,例如:
```
public class PageUtils<T> {
private int currentPage;
private int pageSize;
private int total;
private List<T> data;
// getters and setters
public PageUtils(int currentPage, int pageSize, int total, List<T> data) {
this.currentPage = currentPage;
this.pageSize = pageSize;
this.total = total;
this.data = data;
}
public static <T> PageUtils<T> getPage(int currentPage, int pageSize, List<T> data) {
int total = data.size();
int fromIndex = (currentPage - 1) * pageSize;
int toIndex = fromIndex + pageSize;
if (toIndex > total) {
toIndex = total;
}
List<T> pageData = data.subList(fromIndex, toIndex);
return new PageUtils<>(currentPage, pageSize, total, pageData);
}
}
```
其中,currentPage表示当前页码,pageSize表示每页记录数,total表示总记录数,data表示查询结果。
4. 在Service层调用Mapper接口中的方法,获取查询结果并进行分页处理,例如:
```
@Autowired
private UserMapper userMapper;
public PageUtils<User> findUsers(int currentPage, int pageSize) {
List<User> data = userMapper.findUsers((currentPage - 1) * pageSize, pageSize);
return PageUtils.getPage(currentPage, pageSize, data);
}
```
以上就是自定义分页查询的Mapper的实现步骤,希望对你有所帮助。
阅读全文
相关推荐


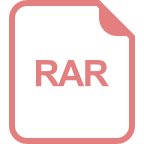
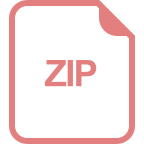












