题目二:模拟书店售书系统 功能需求如下: (1)书籍信息包括:编号、ISBN号、书名、作者、出版社、单价、库存数量。 销售信息包括:日期、ISBN号、数量、单价、总价。 (2)系统功能要求: 1)包括顾客和店员两个用户,顾客只可查询、购买书籍,店员可增、删、改、查、浏览及统计书籍销售情况; 2)书籍信息的增、删、改、查、浏览功能; 3)查询书籍:可ISBN号、书名、作者查询; 4)统计销售情况:输入日期,统计销售金额;输入ISBN号,统计销售金额。 5)书籍销售功能:输入ISBN号,购买数量,显示系统日期、ISBN号、数量、单价、总价,并写入文件。同步更新该书籍的库存数量。 (3)数据组织及存储要求 1)书籍信息、销售信息分别存储在2个文件(.txt)中,增、删、改、查、浏览等操作都是对文件操作,读写文件用多线程实现; 2)书籍、销售的信息在内存中要求使用泛型集合方式组织,具体实现类型自定。 (4)测试数据要求:存储书籍信息及销售信息的两个文件,至少各有10条数据。 (5)难度系数3,使用数据库、算法、前端等知识,可额外加分。 java代码实现
时间: 2023-07-10 11:34:09 浏览: 126
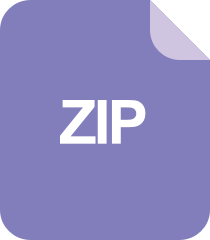
图书销售系统(Java课设)

好的,以下是基于您提供的需求,使用 Java 实现的书店售书系统的代码:
Book.java
```java
public class Book {
private int id;
private String isbn;
private String name;
private String author;
private String publisher;
private double price;
private int stock;
public Book(int id, String isbn, String name, String author, String publisher, double price, int stock) {
this.id = id;
this.isbn = isbn;
this.name = name;
this.author = author;
this.publisher = publisher;
this.price = price;
this.stock = stock;
}
public int getId() {
return id;
}
public String getIsbn() {
return isbn;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public double getPrice() {
return price;
}
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
}
```
Sale.java
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class Sale {
private Date date;
private String isbn;
private int quantity;
private double price;
private double total;
public Sale(String isbn, int quantity, double price) {
this.date = new Date();
this.isbn = isbn;
this.quantity = quantity;
this.price = price;
this.total = quantity * price;
}
public String getDate() {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
return formatter.format(date);
}
public String getIsbn() {
return isbn;
}
public int getQuantity() {
return quantity;
}
public double getPrice() {
return price;
}
public double getTotal() {
return total;
}
public String toString() {
return getDate() + "\t" + isbn + "\t" + quantity + "\t" + price + "\t" + total;
}
}
```
BookStore.java
```java
import java.io.*;
import java.util.*;
public class BookStore {
private List<Book> books;
private List<Sale> sales;
private String bookFile;
private String saleFile;
public BookStore(String bookFile, String saleFile) {
this.books = new ArrayList<>();
this.sales = new ArrayList<>();
this.bookFile = bookFile;
this.saleFile = saleFile;
loadBooks();
loadSales();
}
public void customerMenu() {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("欢迎来到图书店,您是顾客,您可以:");
System.out.println("1. 查询书籍");
System.out.println("2. 购买书籍");
System.out.println("3. 退出");
System.out.print("请输入您的选择:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
searchBooks();
break;
case 2:
buyBook();
break;
case 3:
System.out.println("感谢您的光临,再见!");
return;
default:
System.out.println("无效的选择,请重新输入!");
}
}
}
public void staffMenu() {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("欢迎来到图书店,您是店员,您可以:");
System.out.println("1. 添加书籍");
System.out.println("2. 删除书籍");
System.out.println("3. 修改书籍信息");
System.out.println("4. 查询书籍");
System.out.println("5. 浏览书籍");
System.out.println("6. 统计销售情况");
System.out.println("7. 退出");
System.out.print("请输入您的选择:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
addBook();
break;
case 2:
deleteBook();
break;
case 3:
modifyBook();
break;
case 4:
searchBooks();
break;
case 5:
browseBooks();
break;
case 6:
statistics();
break;
case 7:
System.out.println("感谢您的光临,再见!");
return;
default:
System.out.println("无效的选择,请重新输入!");
}
}
}
public void addBook() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入书籍信息:");
System.out.print("编号:");
int id = scanner.nextInt();
System.out.print("ISBN号:");
String isbn = scanner.next();
System.out.print("书名:");
String name = scanner.next();
System.out.print("作者:");
String author = scanner.next();
System.out.print("出版社:");
String publisher = scanner.next();
System.out.print("单价:");
double price = scanner.nextDouble();
System.out.print("库存数量:");
int stock = scanner.nextInt();
Book book = new Book(id, isbn, name, author, publisher, price, stock);
books.add(book);
saveBooks();
System.out.println("书籍已添加!");
}
public void deleteBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要删除的书籍编号:");
int id = scanner.nextInt();
Book book = findBookById(id);
if (book == null) {
System.out.println("未找到编号为 " + id + " 的书籍!");
} else {
books.remove(book);
saveBooks();
System.out.println("书籍已删除!");
}
}
public void modifyBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要修改的书籍编号:");
int id = scanner.nextInt();
Book book = findBookById(id);
if (book == null) {
System.out.println("未找到编号为 " + id + " 的书籍!");
} else {
System.out.println("请输入要修改的书籍信息:");
System.out.print("ISBN号(原值为 " + book.getIsbn() + "):");
String isbn = scanner.next();
System.out.print("书名(原值为 " + book.getName() + "):");
String name = scanner.next();
System.out.print("作者(原值为 " + book.getAuthor() + "):");
String author = scanner.next();
System.out.print("出版社(原值为 " + book.getPublisher() + "):");
String publisher = scanner.next();
System.out.print("单价(原值为 " + book.getPrice() + "):");
double price = scanner.nextDouble();
System.out.print("库存数量(原值为 " + book.getStock() + "):");
int stock = scanner.nextInt();
book.setIsbn(isbn);
book.setName(name);
book.setAuthor(author);
book.setPublisher(publisher);
book.setPrice(price);
book.setStock(stock);
saveBooks();
System.out.println("书籍已修改!");
}
}
public void searchBooks() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入查询条件:");
System.out.print("1. ISBN号;2. 书名;3. 作者:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("请输入ISBN号:");
String isbn = scanner.next();
Book book = findBookByIsbn(isbn);
if (book == null) {
System.out.println("未找到ISBN号为 " + isbn + " 的书籍!");
} else {
System.out.println("查询结果:");
System.out.println("编号\tISBN号\t书名\t作者\t出版社\t单价\t库存数量");
System.out.println(book.getId() + "\t" + book.getIsbn() + "\t" + book.getName() + "\t" +
book.getAuthor() + "\t" + book.getPublisher() + "\t" + book.getPrice() + "\t" +
book.getStock());
}
break;
case 2:
System.out.print("请输入书名:");
String name = scanner.next();
List<Book> bookList = findBooksByName(name);
if (bookList.isEmpty()) {
System.out.println("未找到书名为 " + name + " 的书籍!");
} else {
System.out.println("查询结果:");
System.out.println("编号\tISBN号\t书名\t作者\t出版社\t单价\t库存数量");
for (Book b : bookList) {
System.out.println(b.getId() + "\t" + b.getIsbn() + "\t" + b.getName() + "\t" +
b.getAuthor() + "\t" + b.getPublisher() + "\t" + b.getPrice() + "\t" +
b.getStock());
}
}
break;
case 3:
System.out.print("请输入作者:");
String author = scanner.next();
bookList = findBooksByAuthor(author);
if (bookList.isEmpty()) {
System.out.println("未找到作者为 " + author + " 的书籍!");
} else {
System.out.println("查询结果:");
System.out.println("编号\tISBN号\t书名\t作者\t出版社\t单价\t库存数量");
for (Book b : bookList) {
System.out.println(b.getId() + "\t" + b.getIsbn() + "\t" + b.getName() + "\t" +
b.getAuthor() + "\t" + b.getPublisher() + "\t" + b.getPrice() + "\t" +
b.getStock());
}
}
break;
default:
System.out.println("无效的选择,请重新输入!");
}
}
public void browseBooks() {
System.out.println("图书馆中共有以下书籍:");
System.out.println("编号\tISBN号\t书名\t作者\t出版社\t单价\t库存数量");
for (Book book : books) {
System.out.println(book.getId() + "\t" + book.getIsbn() + "\t" + book.getName() + "\t" +
book.getAuthor() + "\t" + book.getPublisher() + "\t" + book.getPrice() + "\t" +
book.getStock());
}
}
public void buyBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要购买的书籍的ISBN号:");
String isbn = scanner.next();
Book book = findBookByIsbn(isbn);
if (book == null) {
System.out.println("未找到ISBN号为 " + isbn + " 的书籍!");
} else {
System.out.print("请输入购买数量:");
int quantity = scanner.nextInt();
if (quantity > book.getStock()) {
System.out.println("库存不足!");
} else {
Sale sale = new Sale(isbn, quantity, book.getPrice());
sales.add(sale);
book.setStock(book.getStock() - quantity);
saveSales();
saveBooks();
System.out.println("购买成功!");
System.out.println("系统日期\tISBN号\t数量\t单价\t总价");
System.out.println(sale.toString());
}
}
}
public void statistics() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入统计条件:");
System.out.print("1. 日期;2. ISBN号:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("请输入日期(格式:yyyy-MM-dd):");
String dateStr = scanner.next();
double total = 0;
for (Sale sale : sales) {
if (sale.getDate().equals(dateStr)) {
阅读全文
相关推荐
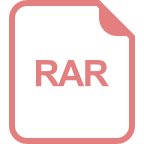

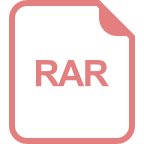
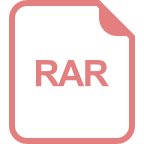
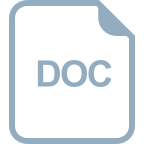
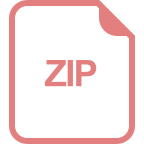
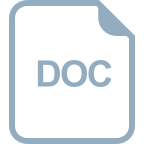
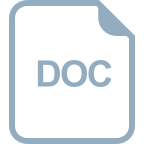
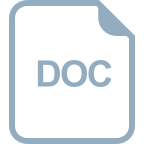
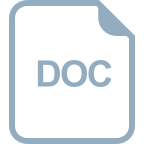
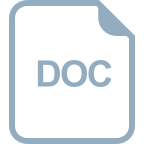
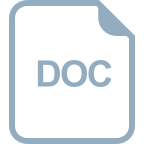
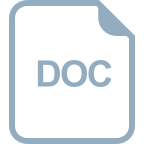
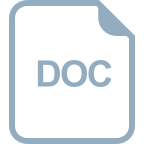
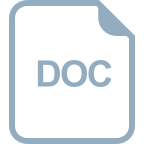
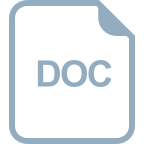