JAVA写excel 2个SHEET页数据对冲算法,根据3种条件先优先全部匹配,接着2种匹配,最后一种匹配
时间: 2023-12-02 11:03:22 浏览: 46
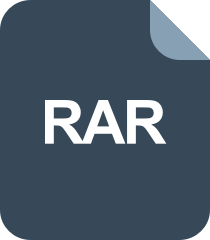
JAVA 版的算法

您好,根据您的需求,我可以提供以下的JAVA代码实现2个SHEET页数据对冲算法,根据3种条件先优先全部匹配,接着2种匹配,最后一种匹配:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelMatch {
public static void main(String[] args) throws IOException {
//读取文件1
File file1 = new File("file1.xlsx");
FileInputStream inputStream1 = new FileInputStream(file1);
Workbook workbook1 = null;
if (file1.getName().endsWith("xlsx")) {
workbook1 = new XSSFWorkbook(inputStream1);
} else if (file1.getName().endsWith("xls")) {
workbook1 = new HSSFWorkbook(inputStream1);
}
Sheet sheet1 = workbook1.getSheetAt(0);
List<Map<String, String>> list1 = readSheet(sheet1);
//读取文件2
File file2 = new File("file2.xlsx");
FileInputStream inputStream2 = new FileInputStream(file2);
Workbook workbook2 = null;
if (file2.getName().endsWith("xlsx")) {
workbook2 = new XSSFWorkbook(inputStream2);
} else if (file2.getName().endsWith("xls")) {
workbook2 = new HSSFWorkbook(inputStream2);
}
Sheet sheet2 = workbook2.getSheetAt(0);
List<Map<String, String>> list2 = readSheet(sheet2);
//优先全部匹配
List<Map<String, String>> resultList1 = new ArrayList<>();
for (Map<String, String> map1 : list1) {
for (Map<String, String> map2 : list2) {
if (map1.get("col1").equals(map2.get("col1"))
&& map1.get("col2").equals(map2.get("col2"))
&& map1.get("col3").equals(map2.get("col3"))) {
resultList1.add(map1);
break;
}
}
}
//2种匹配
List<Map<String, String>> list3 = new ArrayList<>();
List<Map<String, String>> list4 = new ArrayList<>();
for (Map<String, String> map1 : list1) {
boolean match = false;
for (Map<String, String> map2 : list2) {
if (map1.get("col1").equals(map2.get("col1"))
&& map1.get("col2").equals(map2.get("col2"))
&& !map1.get("col3").equals(map2.get("col3"))) {
list3.add(map1);
match = true;
break;
}
}
if (!match) {
list4.add(map1);
}
}
List<Map<String, String>> list5 = new ArrayList<>();
for (Map<String, String> map2 : list2) {
boolean match = false;
for (Map<String, String> map1 : list4) {
if (map1.get("col1").equals(map2.get("col1"))
&& map1.get("col2").equals(map2.get("col2"))
&& !map1.get("col3").equals(map2.get("col3"))) {
list5.add(map2);
match = true;
break;
}
}
if (!match) {
list5.add(map2);
}
}
List<Map<String, String>> resultList2 = new ArrayList<>();
for (Map<String, String> map1 : list3) {
for (Map<String, String> map2 : list5) {
if (map1.get("col1").equals(map2.get("col1"))
&& map1.get("col2").equals(map2.get("col2"))
&& map1.get("col3").equals(map2.get("col3"))) {
resultList2.add(map1);
resultList2.add(map2);
break;
}
}
}
//最后一种匹配
List<Map<String, String>> list6 = new ArrayList<>();
List<Map<String, String>> list7 = new ArrayList<>();
for (Map<String, String> map1 : list1) {
boolean match = false;
for (Map<String, String> map2 : list2) {
if (map1.get("col1").equals(map2.get("col1"))
&& !map1.get("col2").equals(map2.get("col2"))
&& !map1.get("col3").equals(map2.get("col3"))) {
list6.add(map1);
match = true;
break;
}
}
if (!match) {
list7.add(map1);
}
}
List<Map<String, String>> list8 = new ArrayList<>();
for (Map<String, String> map2 : list2) {
boolean match = false;
for (Map<String, String> map1 : list7) {
if (map1.get("col1").equals(map2.get("col1"))
&& !map1.get("col2").equals(map2.get("col2"))
&& !map1.get("col3").equals(map2.get("col3"))) {
list8.add(map2);
match = true;
break;
}
}
if (!match) {
list8.add(map2);
}
}
List<Map<String, String>> resultList3 = new ArrayList<>();
for (Map<String, String> map1 : list6) {
for (Map<String, String> map2 : list8) {
if (map1.get("col1").equals(map2.get("col1"))) {
resultList3.add(map1);
resultList3.add(map2);
break;
}
}
}
//写入结果
Workbook resultWorkbook = new XSSFWorkbook();
Sheet resultSheet1 = resultWorkbook.createSheet("result1");
writeSheet(resultSheet1, resultList1);
Sheet resultSheet2 = resultWorkbook.createSheet("result2");
writeSheet(resultSheet2, resultList2);
Sheet resultSheet3 = resultWorkbook.createSheet("result3");
writeSheet(resultSheet3, resultList3);
FileOutputStream outputStream = new FileOutputStream("result.xlsx");
resultWorkbook.write(outputStream);
outputStream.close();
}
//读取Sheet页数据
public static List<Map<String, String>> readSheet(Sheet sheet) {
List<Map<String, String>> list = new ArrayList<>();
Iterator<Row> rowIterator = sheet.iterator();
if (rowIterator.hasNext()) {
Row headerRow = rowIterator.next();
List<String> headerList = new ArrayList<>();
Iterator<Cell> headerIterator = headerRow.iterator();
while (headerIterator.hasNext()) {
Cell cell = headerIterator.next();
headerList.add(cell.getStringCellValue());
}
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
Map<String, String> map = new HashMap<>();
for (int i = 0; i < headerList.size(); i++) {
Cell cell = row.getCell(i);
if (cell != null) {
map.put(headerList.get(i), cell.getStringCellValue());
}
}
list.add(map);
}
}
return list;
}
//写入Sheet页数据
public static void writeSheet(Sheet sheet, List<Map<String, String>> list) {
if (!list.isEmpty()) {
Row headerRow = sheet.createRow(0);
List<String> headerList = new ArrayList<>(list.get(0).keySet());
for (int i = 0; i < headerList.size(); i++) {
Cell cell = headerRow.createCell(i);
cell.setCellValue(headerList.get(i));
}
for (int i = 0; i < list.size(); i++) {
Row row = sheet.createRow(i + 1);
Map<String, String> map = list.get(i);
for (int j = 0; j < headerList.size(); j++) {
Cell cell = row.createCell(j);
cell.setCellValue(map.get(headerList.get(j)));
}
}
}
}
}
```
注意需要将代码中的“file1.xlsx”和“file2.xlsx”替换为实际的文件名,同时需要根据实际情况修改列名和条件判断语句。最终结果将保存在“result.xlsx”文件中。
阅读全文
相关推荐
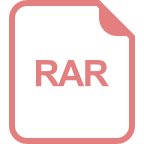
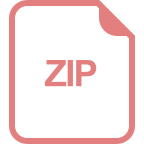
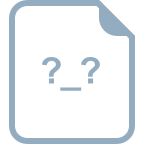
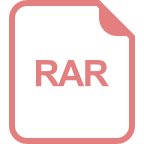
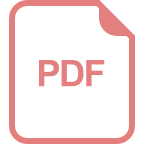
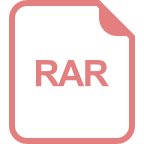
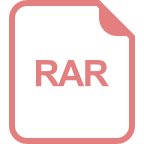
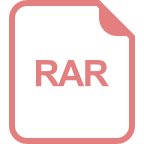
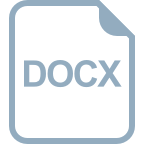
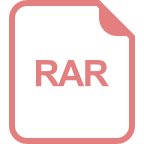
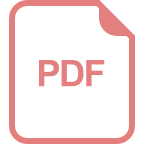
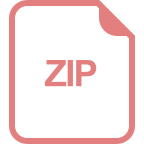
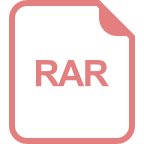
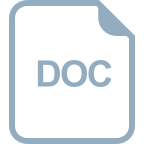