可以远程调用另一个进程内的函数吗
时间: 2023-11-19 22:05:30 浏览: 36
是的,可以使用远程过程调用(RPC)技术来实现在不同进程之间调用函数的功能。RPC技术允许你像调用本地函数一样调用远程函数,RPC系统会自动处理底层的进程间通信细节。
在Qt中,可以使用Qt Remote Objects框架来实现RPC。Qt Remote Objects通过自动生成远程接口的代码来简化RPC的开发,并提供了一种方便的方式来编写跨平台的RPC应用程序。
Qt Remote Objects使用Qt的元对象系统来定义远程接口。开发人员只需要在类的定义中添加Q_INVOKABLE宏即可将类的方法暴露为远程调用接口。然后使用Qt的moc工具生成远程接口的代码,这些代码可以被客户端和服务器端使用。
以下是一个简单的示例,展示了如何在Qt中使用Qt Remote Objects来实现跨进程的函数调用:
```cpp
// MyRemoteObject.h
#include <QObject>
class MyRemoteObject : public QObject
{
Q_OBJECT
public:
Q_INVOKABLE int add(int a, int b);
};
// MyRemoteObject.cpp
#include "MyRemoteObject.h"
int MyRemoteObject::add(int a, int b)
{
return a + b;
}
// Server.cpp
#include <QCoreApplication>
#include <QtRemoteObjects>
#include "MyRemoteObject.h"
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
MyRemoteObject myRemoteObject;
QRemoteObjectRegistry::setHostUrl("local:registry");
QRemoteObjectRegistry::globalRegistry()->registerObject(&myRemoteObject, "myRemoteObject");
return app.exec();
}
// Client.cpp
#include <QCoreApplication>
#include <QtRemoteObjects>
#include <QDebug>
#include "MyRemoteObject.h"
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
auto myRemoteObject = QRemoteObjectNode::waitForRemoteObject<MyRemoteObject>("myRemoteObject", QUrl("local:registry"));
if (myRemoteObject) {
int result = myRemoteObject->add(1, 2);
qDebug() << "Result:" << result;
}
return app.exec();
}
```
在上面的示例中,我们定义了一个简单的MyRemoteObject类,并将其方法add()暴露为远程调用接口。在服务器端,我们创建了一个MyRemoteObject实例并将其注册到远程对象注册表中。在客户端,我们使用QRemoteObjectNode::waitForRemoteObject()方法获取MyRemoteObject的远程代理,并使用代理调用add()方法。
需要注意的是,Qt Remote Objects使用了一些高级的C++特性,如元对象系统和信号槽机制。因此,需要使用Qt的元对象编译器(moc)来生成远程接口的代码。另外,Qt Remote Objects的使用需要在编译和链接时添加特定的选项。
相关推荐
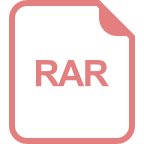
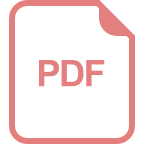














