编写爬虫程序,获取https://www.chzc.edu.cn/info/1024/66328.htm对应网页中信息
时间: 2023-09-07 09:13:27 浏览: 90
好的,我可以帮你编写一个Python爬虫程序来获取该网页中的信息。你需要安装requests和BeautifulSoup库,可以使用以下命令来安装:
```
pip install requests
pip install beautifulsoup4
```
然后你可以使用以下代码来实现:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.chzc.edu.cn/info/1024/66328.htm'
res = requests.get(url)
res.encoding = 'utf-8'
soup = BeautifulSoup(res.text, 'html.parser')
title = soup.select('.title')[0].text.strip() # 获取标题
content = soup.select('#vsb_content')[0].text.strip() # 获取内容
print(title)
print(content)
```
这段代码发送一个GET请求到该网页,然后使用BeautifulSoup库解析HTML文档,从中提取标题和内容。最后打印出来。请注意,这段代码仅供学习和参考,未经网站所有者的允许,请勿在未经授权的情况下爬取网站数据。
相关问题
使用python爬虫爬取“https://www.chzc.edu.cn/info/1024/66328.htm”中图片
好的,可以使用Python的requests和BeautifulSoup库来实现爬取图片的功能。以下是实现的代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.chzc.edu.cn/info/1024/66328.htm"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
# 找到所有的图片标签
img_tags = soup.find_all("img")
# 遍历图片标签并下载图片
for img_tag in img_tags:
img_url = img_tag["src"]
img_name = img_url.split("/")[-1]
img_response = requests.get(img_url)
with open(img_name, "wb") as f:
f.write(img_response.content)
```
解释一下代码:
首先,我们使用requests库获取网页的HTML代码。然后,使用BeautifulSoup库对HTML代码进行解析。
接着,我们使用find_all方法找到所有的图片标签。在这个例子中,该网页只有一张图片,所以img_tags列表中只有一个元素。
最后,我们遍历图片标签并使用requests库下载图片。图片的文件名可以通过图片URL中的最后一个斜杠后的字符串获得。图片保存在当前目录下。
希望这个代码可以帮到你。
使用python爬虫爬取“https://www.chzc.edu.cn/info/1024/66328.htm”任一信息代码
好的,以下是使用Python爬虫爬取该链接信息的代码示例:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.chzc.edu.cn/info/1024/66328.htm"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/81.0.4044.138 Safari/537.36"
}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, "html.parser")
# 获取文章标题
title = soup.find("h2", class_="contentTitle").text.strip()
print("文章标题:", title)
# 获取文章正文
content = soup.find("div", class_="contentInfo").text.strip()
print("文章正文:", content)
```
运行上述代码,即可获取该链接中的文章标题和正文。需要注意的是,为了避免触发网站的反爬机制,我们在请求头中添加了User-Agent信息,模拟浏览器访问。
阅读全文
相关推荐





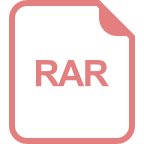
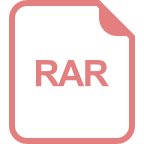
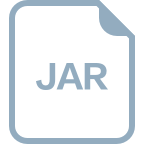
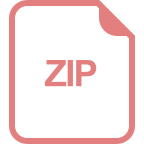
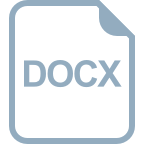