c语言读取文本文件中学生成绩数据并按姓氏排序存入链表
时间: 2023-05-22 16:06:45 浏览: 136
可以使用文件操作函数fopen、fscanf和fclose来读取文本文件中的学生成绩数据并存入链表。具体实现可以参考下面的代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生信息结构体
typedef struct {
char name[20]; // 学生姓名
int score; // 学生成绩
} Student;
// 定义链表节点结构体
typedef struct Node {
Student data; // 数据域
struct Node* next; // 指针域
} Node;
// 插入链表节点
void insert(Node** head, Student data) {
Node* p = (Node*) malloc(sizeof(Node));
p->data = data;
p->next = NULL;
if (*head == NULL) {
*head = p;
} else {
Node* q = *head;
Node* prev = NULL;
while (q && strcmp(q->data.name, data.name) < 0) {
prev = q;
q = q->next;
}
if (prev == NULL) {
p->next = *head;
*head = p;
} else {
p->next = q;
prev->next = p;
}
}
}
// 遍历链表并打印数据
void print(Node* head) {
Node* p = head;
while (p) {
printf("%s %d\n", p->data.name, p->data.score);
p = p->next;
}
}
int main() {
FILE* fp = fopen("scores.txt", "r");
if (!fp) {
printf("读取文件失败!\n");
return -1;
}
Node* head = NULL;
char name[20];
int score;
while (fscanf(fp, "%s%d", name, &score) == 2) {
Student s;
strcpy(s.name, name);
s.score = score;
insert(&head, s);
}
fclose(fp);
print(head);
return 0;
}
```
该代码可以读取名为"scores.txt"的文本文件中的学生成绩数据,并按照学生姓名的字典序排序存入链表,最后打印出所有学生的姓名和成绩。
阅读全文
相关推荐
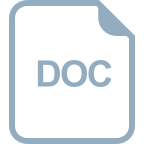
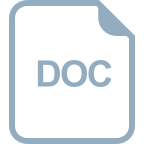
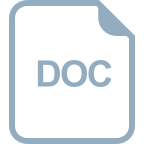
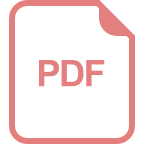
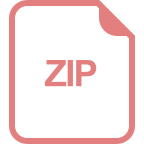
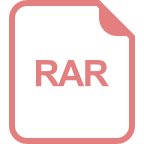
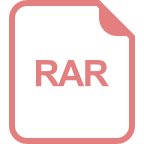
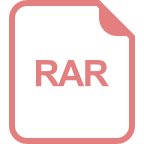
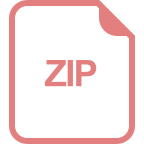
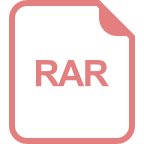
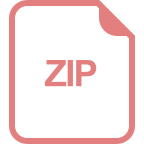
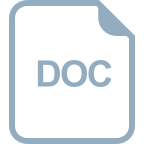
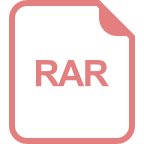
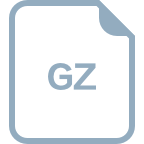