unity功夫荣耀代码
时间: 2023-07-03 12:17:07 浏览: 96
很抱歉,我无法提供完整的Unity功夫荣耀代码,因为这需要根据具体的游戏设计和开发需求进行编写和修改。不过,以下是一些常用的代码片段和示例,供你参考和学习:
1. 角色移动控制
```csharp
public class PlayerController : MonoBehaviour {
public float speed = 6.0f;
public float jumpSpeed = 8.0f;
public float gravity = 20.0f;
private Vector3 moveDirection = Vector3.zero;
private CharacterController controller;
private bool isGrounded = false;
void Start() {
controller = GetComponent<CharacterController>();
}
void Update() {
if (controller.isGrounded) {
isGrounded = true;
moveDirection = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical"));
moveDirection = transform.TransformDirection(moveDirection);
moveDirection *= speed;
if (Input.GetButton("Jump")) {
moveDirection.y = jumpSpeed;
}
}
moveDirection.y -= gravity * Time.deltaTime;
controller.Move(moveDirection * Time.deltaTime);
}
}
```
2. 武器攻击控制
```csharp
public class WeaponController : MonoBehaviour {
public float damage = 10.0f;
public float hitRange = 1.5f;
public float attackSpeed = 1.0f;
private bool canAttack = true;
void Start() {
}
void Update() {
if (canAttack && Input.GetMouseButtonDown(0)) {
StartCoroutine(Attack());
}
}
IEnumerator Attack() {
canAttack = false;
RaycastHit hit;
if (Physics.Raycast(transform.position, transform.forward, out hit, hitRange)) {
if (hit.transform.CompareTag("Enemy")) {
hit.transform.GetComponent<EnemyController>().TakeDamage(damage);
}
}
yield return new WaitForSeconds(attackSpeed);
canAttack = true;
}
}
```
3. 敌人AI控制
```csharp
public class EnemyController : MonoBehaviour {
public float health = 100.0f;
public float attackRange = 2.0f;
public float attackSpeed = 1.0f;
private Transform target;
private bool canAttack = true;
void Start() {
target = GameObject.FindGameObjectWithTag("Player").transform;
}
void Update() {
float distance = Vector3.Distance(transform.position, target.position);
if (distance < attackRange) {
if (canAttack) {
StartCoroutine(Attack());
}
} else {
transform.LookAt(target);
transform.Translate(Vector3.forward * Time.deltaTime);
}
}
IEnumerator Attack() {
canAttack = false;
target.GetComponent<PlayerController>().TakeDamage();
yield return new WaitForSeconds(attackSpeed);
canAttack = true;
}
public void TakeDamage(float damage) {
health -= damage;
if (health <= 0) {
Die();
}
}
void Die() {
Destroy(gameObject);
}
}
```
以上代码片段和示例可以帮助你了解Unity功夫荣耀中常用的代码结构和语法,但是具体的实现方式需要根据游戏设计和开发需求进行编写和修改。
阅读全文
相关推荐










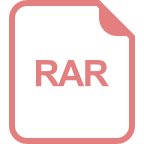