补充函数 sortByChoose()的剩余部分,其要求是利用选择排序算法根据学生成绩(score)实现对结构体数组元素降序排序。 函数接口定义: void sortByChoose(struct Student *pData,int n); 裁判测试程序样例: #include<stdio.h> #include<stdlib.h> #define N 10 struct Student { int num; int score; }; void sortByChoose(s
时间: 2024-03-01 08:49:19 浏览: 36
ort(struct Student *pData, int n) {
int i, j, maxIndex;
struct Student temp;
for (i = 0; i < n - 1; i++) {
maxIndex = i;
for (j = i + 1; j < n; j++) {
if (pData[j].score > pData[maxIndex].score) {
maxIndex = j;
}
}
if (maxIndex != i) {
temp = pData[i];
pData[i] = pData[maxIndex];
pData[maxIndex] = temp;
}
}
}
相关问题
题:补充函数 sortByChoose()的剩余部分,其要求是利用选择排序算法根据学生成绩(score)实现对结构体数组元素降序排序。
以下是 `sortByChoose()` 函数的代码实现:
```c++
void sortByChoose(student stu[], int n) {
for (int i = 0; i < n - 1; i++) {
int maxIndex = i;
for (int j = i + 1; j < n; j++) {
if (stu[j].score > stu[maxIndex].score) {
maxIndex = j;
}
}
if (maxIndex != i) {
swap(stu[i], stu[maxIndex]);
}
}
}
```
该函数利用了选择排序算法,通过遍历数组中的所有元素,每次找到剩余元素中分数最高的学生,然后将其与当前元素进行交换,从而实现对学生成绩的降序排序。
需要注意的是,该函数接收一个结构体数组 `stu` 和数组长度 `n` 作为参数。结构体数组中的每个元素包含了学生的姓名和分数信息。在函数中,我们使用 `i` 和 `j` 两个变量分别表示当前遍历到的元素下标和剩余元素中最高分数的元素下标。在内层循环中,如果发现有比当前最高分数更高的学生,则更新 `maxIndex` 的值。最后,如果 `maxIndex` 不等于 `i`,说明找到了一个更高分数的学生,需要将其与当前元素进行交换。
使用该函数示例:
```c++
#include <iostream>
#include <string>
using namespace std;
struct student {
string name;
int score;
};
void sortByChoose(student stu[], int n);
int main() {
student stu[] = {
{"张三", 80},
{"李四", 90},
{"王五", 70},
{"赵六", 85},
{"钱七", 95}
};
int n = sizeof(stu) / sizeof(student);
sortByChoose(stu, n);
for (int i = 0; i < n; i++) {
cout << stu[i].name << " " << stu[i].score << endl;
}
return 0;
}
// 输出结果:
// 钱七 95
// 李四 90
// 赵六 85
// 张三 80
// 王五 70
```
上述代码中,我们定义了一个结构体 `student`,包含了学生的姓名和分数信息。在 `main()` 函数中,我们定义了一个结构体数组 `stu`,其中包含了 5 名学生的信息。然后调用 `sortByChoose()` 函数进行降序排序,并输出排序后的结果。
c语言编程:利用“选择排序算法“对结构体数组进行排序补充函数 sortByChoose()的剩余部分,其要求是利用选择排序算法根据学生成绩(score)实现对结构体数组元素降序排序。 函数接口定义: void sortByChoose(struct Student *pData,int n);
好的,我会为你补充函数sortByChoose()的剩余部分。以下是完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Student {
char name[20];
int score;
};
void sortByChoose(struct Student *pData, int n) {
int i, j, max_index;
struct Student temp;
for (i = 0; i < n - 1; i++) {
max_index = i;
for (j = i + 1; j < n; j++) {
if (pData[j].score > pData[max_index].score) {
max_index = j;
}
}
if (max_index != i) {
temp = pData[i];
pData[i] = pData[max_index];
pData[max_index] = temp;
}
}
}
int main() {
struct Student stu[5] = {
{"Tom", 89},
{"Jerry", 76},
{"Mike", 92},
{"Mary", 85},
{"John", 78}
};
int i;
printf("Before sorting:\n");
for (i = 0; i < 5; i++) {
printf("%s: %d\n", stu[i].name, stu[i].score);
}
sortByChoose(stu, 5);
printf("\nAfter sorting:\n");
for (i = 0; i < 5; i++) {
printf("%s: %d\n", stu[i].name, stu[i].score);
}
return 0;
}
```
函数sortByChoose()使用选择排序算法,对结构体数组中的元素按照学生成绩(score)进行降序排序。在函数中,我们定义了三个变量:i、j和max_index。i和j用于循环遍历数组,max_index用于存储当前最大值的下标。我们首先将max_index初始化为i,然后在内层循环中查找最大值的下标,并将其存储在max_index中。如果max_index不等于i,说明第i个元素不是当前最大值,需要将其与最大值交换位置。最后,函数执行完毕后,结构体数组stu中的元素就已经按照学生成绩降序排列了。
我希望这个代码能够帮助到你,如果你有任何问题,请随时问我!
相关推荐
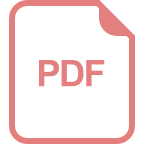
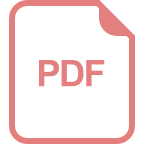
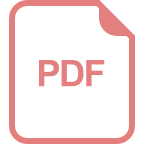
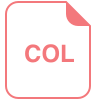
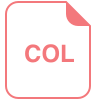
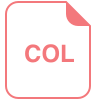
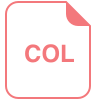
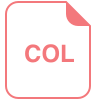

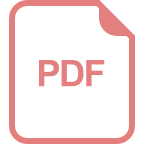
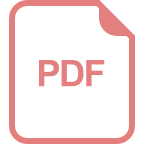
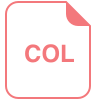
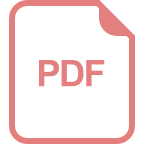
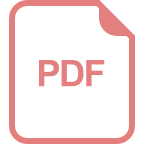
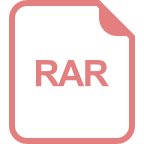
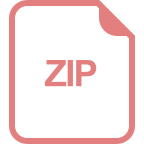
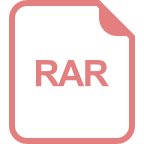