现在你有一个数据集,你需要使用XGBoost算法进行回归预测,请告诉我具体的代码以及XGBoost对参数调优的过程代码,并绘制出曲线
时间: 2023-08-31 16:33:13 浏览: 60
### 回答1:
首先,需要导入所需的库和数据集,以及将数据集划分为训练集和测试集:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from xgboost import XGBRegressor
from sklearn.metrics import mean_squared_error
# 导入数据集
data = pd.read_csv('data.csv')
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data.drop('target', axis=1), data['target'], test_size=0.2, random_state=42)
```
接下来,使用XGBoost算法进行回归预测:
```python
# 定义XGBoost模型
xgb = XGBRegressor()
# 在训练集上训练模型
xgb.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = xgb.predict(X_test)
# 计算均方误差
mse = mean_squared_error(y_test, y_pred)
print('均方误差:', mse)
```
XGBoost对参数调优的过程代码如下:
```python
from sklearn.model_selection import GridSearchCV
# 定义参数范围
param_grid = {
'max_depth': [3, 4, 5],
'learning_rate': [0.01, 0.1, 0.5],
'n_estimators': [50, 100, 200]
}
# 定义模型
xgb = XGBRegressor()
# 使用网格搜索进行参数调优
grid_search = GridSearchCV(xgb, param_grid=param_grid, scoring='neg_mean_squared_error', cv=5, n_jobs=-1)
grid_search.fit(X_train, y_train)
# 输出最优参数
print('最优参数:', grid_search.best_params_)
```
最后,绘制出XGBoost模型的学习曲线,以评估模型的性能:
```python
import matplotlib.pyplot as plt
from sklearn.model_selection import learning_curve
# 定义函数绘制学习曲线
def plot_learning_curve(estimator, title, X, y, ylim=None, cv=None, n_jobs=None, train_sizes=np.linspace(0.1, 1.0, 5)):
plt.figure()
plt.title(title)
if ylim is not None:
plt.ylim(*ylim)
plt.xlabel("Training examples")
plt.ylabel("Score")
train_sizes, train_scores, test_scores = learning_curve(estimator, X, y, cv=cv, n_jobs=n_jobs, train_sizes=train_sizes, scoring='neg_mean_squared_error')
train_scores_mean = -np.mean(train_scores, axis=1)
train_scores_std = np.std(train_scores, axis=1)
test_scores_mean = -np.mean(test_scores, axis=1)
test_scores_std = np.std(test_scores, axis=1)
plt.grid()
plt.fill_between(train_sizes, train_scores_mean - train_scores_std, train_scores_mean + train_scores_std, alpha=0.1, color="r")
plt.fill_between(train_sizes, test_scores_mean - test_scores_std, test_scores_mean + test_scores_std, alpha=0.1, color="g")
plt.plot(train_sizes, train_scores_mean, 'o-', color="r", label="Training score")
plt.plot(train_sizes, test_scores_mean, 'o-', color="g", label="Cross-validation score")
plt.legend(loc="best")
return plt
# 绘制学习曲线
plot_learning_curve(grid_search.best_estimator_, 'Learning Curve', X_train, y_train, cv=5, n_jobs=-1)
plt.show()
```
以上就是使用XGBoost算法进行回归预测以及对参数调优的过程代码,并绘制出的学习曲线。
### 回答2:
使用XGBoost算法进行回归预测可以按照以下步骤进行:
1. 导入所需的库和模块:
```python
import xgboost as xgb
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import matplotlib.pyplot as plt
```
2. 加载数据集并划分训练集和测试集:
```python
# 假设数据集特征保存在X中,标签保存在Y中
X_train, X_test, y_train, y_test = train_test_split(X, Y, test_size=0.2, random_state=42)
```
3. 创建XGBoost回归模型:
```python
model = xgb.XGBRegressor()
```
4. 调优参数并训练模型:
```python
# 简单调优示例,可根据具体情况进行调整
param_dict = {'max_depth': [3, 5, 7], 'learning_rate': [0.1, 0.01, 0.001], 'n_estimators': [100, 200, 300]}
best_rmse = float('inf')
best_param = None
for max_depth in param_dict['max_depth']:
for learning_rate in param_dict['learning_rate']:
for n_estimators in param_dict['n_estimators']:
model.set_params(max_depth=max_depth, learning_rate=learning_rate, n_estimators=n_estimators)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
rmse = np.sqrt(mean_squared_error(y_test, y_pred))
if rmse < best_rmse:
best_rmse = rmse
best_param = {'max_depth': max_depth, 'learning_rate': learning_rate, 'n_estimators': n_estimators}
```
5. 使用最优参数重新训练模型并绘制曲线:
```python
model.set_params(**best_param)
model.fit(X_train, y_train)
# 绘制特征重要度曲线
fig, ax = plt.subplots(figsize=(10, 8))
xgb.plot_importance(model, ax=ax)
plt.show()
```
以上是使用XGBoost算法进行回归预测的代码示例,具体的参数调优过程可以根据实际情况调整参数范围和调优策略。
### 回答3:
首先,我们需要导入所需的库和数据。假设我们的数据集为`data.csv`,其中包含了特征和目标变量。
import pandas as pd
import xgboost as xgb
import matplotlib.pyplot as plt
# 读取数据集
data = pd.read_csv('data.csv')
接下来,我们需要将数据集划分为特征矩阵X和目标向量y。
# 将特征和目标变量分离
X = data.drop('target', axis=1)
y = data['target']
然后,我们可以定义XGBoost回归模型并进行参数调优。
# 定义XGBoost回归模型
model = xgb.XGBRegressor()
# 定义参数调优范围
params = {
'learning_rate': [0.01, 0.1, 0.5],
'max_depth': [3, 5, 7],
'n_estimators': [100, 500, 1000]
}
# 使用GridSearchCV进行参数调优
from sklearn.model_selection import GridSearchCV
grid_search = GridSearchCV(estimator=model, param_grid=params, scoring='neg_mean_squared_error', cv=3)
grid_search.fit(X, y)
# 输出最佳参数
print(grid_search.best_params_)
最后,我们可以绘制出学习曲线和验证曲线来评估模型的性能和参数调优的效果。
# 获取每个参数对应的得分
train_scores = grid_search.cv_results_['mean_train_score']
test_scores = grid_search.cv_results_['mean_test_score']
# 获取参数的变化情况
param_values = [str(x) for x in grid_search.param_grid.values()]
# 绘制学习曲线
plt.figure(figsize=(12, 6))
plt.plot(param_values, train_scores, label='Train')
plt.plot(param_values, test_scores, label='Test')
plt.xlabel('Parameters')
plt.ylabel('Mean Squared Error')
plt.legend()
plt.title('Learning Curve')
plt.show()
通过学习曲线和验证曲线的对比,我们可以选择具有较低均方误差的参数组合作为最终的模型参数。
相关推荐
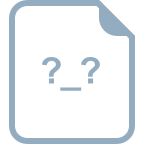
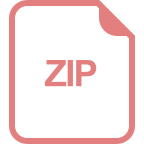
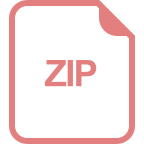














