写一段运用到jdbc的学生管理系统代码,包括学生姓名,年龄和随机的考试成绩
时间: 2024-06-08 07:09:26 浏览: 89
以下是一个简单的学生管理系统代码,使用 JDBC 连接数据库进行数据的插入、查询和展示。
```java
import java.sql.*;
public class StudentManagementSystem {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 连接 MySQL 数据库
Class.forName("com.mysql.cj.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/school";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
stmt = conn.createStatement();
// 创建 student 表
String createTableSql = "CREATE TABLE IF NOT EXISTS student (id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(20), age INT, score FLOAT)";
stmt.executeUpdate(createTableSql);
// 插入学生数据
String insertSql = "INSERT INTO student (name, age, score) VALUES (?, ?, ?)";
PreparedStatement ps = conn.prepareStatement(insertSql);
String[] names = {"Alice", "Bob", "Charlie", "David"};
int[] ages = {20, 21, 22, 23};
for (int i = 0; i < 4; i++) {
ps.setString(1, names[i]);
ps.setInt(2, ages[i]);
ps.setFloat(3, (float) (Math.random() * 100));
ps.executeUpdate();
}
// 查询所有学生数据
String selectSql = "SELECT * FROM student";
rs = stmt.executeQuery(selectSql);
// 展示查询结果
System.out.println("学生列表:");
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
float score = rs.getFloat("score");
System.out.printf("%d\t%s\t%d\t%.2f\n", id, name, age, score);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
}
```
在该示例代码中,我们首先连接到 MySQL 数据库,然后创建一个名为 `student` 的表,在表中插入学生数据,最后查询并展示所有学生的信息。其中,学生表包含四个字段:id、name、age 和 score,分别表示学生的编号、姓名、年龄和成绩。通过 JDBC API,我们可以方便地进行 SQL 操作,包括数据的插入、更新、删除和查询等。
阅读全文
相关推荐
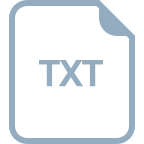
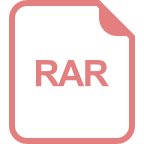
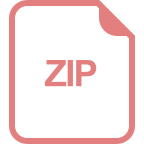
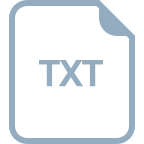
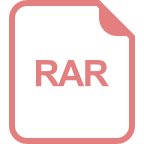
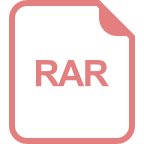
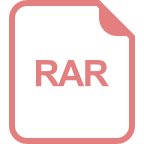
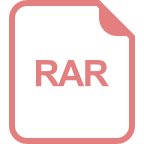
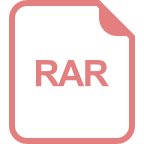
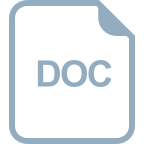
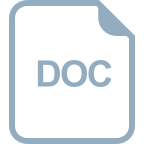
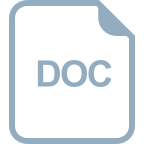
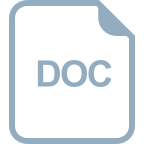
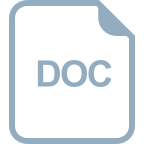
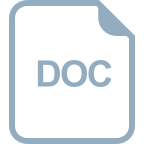
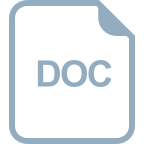
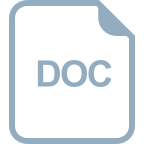