用C语言分别用递归和非递归方法实现链表逆置
时间: 2024-05-13 15:20:53 浏览: 107
递归方法实现链表逆置:
```c
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* reverseList(struct ListNode* head) {
if (head == NULL || head->next == NULL) { // 链表为空或只有一个节点
return head;
}
struct ListNode *newNode = reverseList(head->next); // 递归反转子链表
head->next->next = head; // 修改指针指向
head->next = NULL;
return newNode;
}
```
非递归方法实现链表逆置:
```c
struct ListNode* reverseList(struct ListNode* head) {
if (head == NULL || head->next == NULL) { // 链表为空或只有一个节点
return head;
}
struct ListNode *prev = NULL, *cur = head, *next = head->next;
while (cur != NULL) {
cur->next = prev; // 修改指针指向
prev = cur;
cur = next;
if (next != NULL) {
next = next->next;
}
}
return prev;
}
```
阅读全文
相关推荐
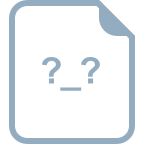
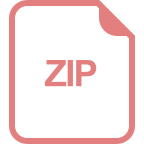
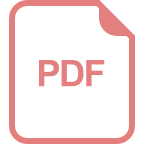
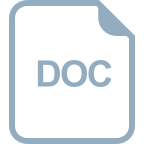



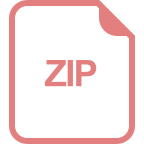
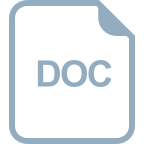
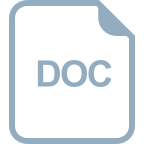
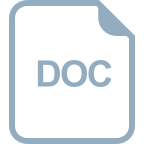





