请将我的数据集里面的3D图像变成2D、三通道、png格式的图像(要求调整窗宽和窗位,以突出所需区域的细节)。数据集里面的3D标签变成(要求背景灰度值0、肝脏灰度值1、肝脏肿瘤灰度值2的)2D的png格式标签。 LiTS2017数据集里面包含Training Batch 1和Training Batch 2两个文件夹。Training Batch 1文件夹里面有图像,图像的拓展名为.nii。Training Batch 2文件夹里面有标签,标签拓展名为.nii。 LiTS2017数据集的路径为C:\Users\Administrator\Desktop\LiTS2017。 保存路径为C:\Users\Administrator\Desktop\2D-LiTS2017,请帮我创建好对应文件夹,并且将转换好的数据保存到对应的文件夹。
时间: 2024-02-03 08:13:43 浏览: 271

mnist数据集转为png格式图片

好的,你需要使用Python中的SimpleITK库来读取和处理nii文件,并使用OpenCV库将3通道的图像转换为2D的三通道png格式的图像。以下是代码实现:
```python
import os
import SimpleITK as sitk
import cv2
# 定义窗宽和窗位
WW = 400
WL = 50
# 定义输入路径和输出路径
input_path = r'C:\Users\Administrator\Desktop\LiTS2017'
output_path = r'C:\Users\Administrator\Desktop\2D-LiTS2017'
# 创建输出文件夹
if not os.path.exists(output_path):
os.makedirs(output_path)
os.makedirs(os.path.join(output_path, 'image'))
os.makedirs(os.path.join(output_path, 'label'))
# 读取nii文件并转换为png格式保存
for folder in ['Training Batch 1', 'Training Batch 2']:
print('Processing', folder)
input_folder = os.path.join(input_path, folder)
output_folder_image = os.path.join(output_path, 'image', folder)
output_folder_label = os.path.join(output_path, 'label', folder)
if not os.path.exists(output_folder_image):
os.makedirs(output_folder_image)
if not os.path.exists(output_folder_label):
os.makedirs(output_folder_label)
for file in os.listdir(input_folder):
if file.endswith('.nii'):
print('Processing', file)
# 读取nii文件
itk_img = sitk.ReadImage(os.path.join(input_folder, file))
img_array = sitk.GetArrayFromImage(itk_img)
origin = itk_img.GetOrigin()
spacing = itk_img.GetSpacing()
direction = itk_img.GetDirection()
# 调整窗宽和窗位
img_array = (img_array - WL + WW/2) / WW * 255
img_array[img_array < 0] = 0
img_array[img_array > 255] = 255
# 将3D图像转换为2D的三通道png格式的图像
for i in range(img_array.shape[0]):
img = img_array[i, :, :]
img = cv2.merge([img, img, img])
img = img.astype('uint8')
filename = os.path.splitext(file)[0] + '_' + str(i+1).zfill(3) + '.png'
cv2.imwrite(os.path.join(output_folder_image, filename), img)
# 读取对应的标签文件
label_file = file.replace('volume', 'segmentation')
itk_label = sitk.ReadImage(os.path.join(input_folder, label_file))
label_array = sitk.GetArrayFromImage(itk_label)
# 将3D标签转换为2D的png格式的标签
for i in range(label_array.shape[0]):
label = label_array[i, :, :]
label[label == 1] = 128
label[label == 2] = 255
filename = os.path.splitext(label_file)[0] + '_' + str(i+1).zfill(3) + '.png'
cv2.imwrite(os.path.join(output_folder_label, filename), label)
print('Done!')
```
这段代码会在C:\Users\Administrator\Desktop\2D-LiTS2017下创建两个文件夹:image和label,分别存放转换后的图像和标签。其中,每个nii文件将会转换成若干个png格式的图像和标签,每个文件名中包含了对应的体积和切片编号。
阅读全文
相关推荐
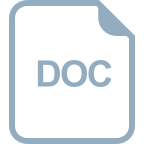
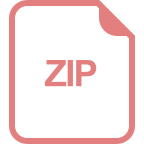






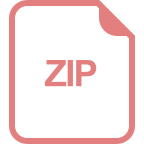
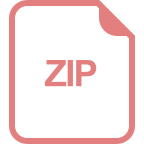
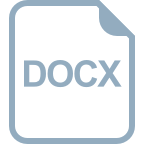
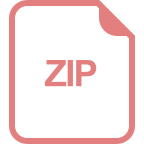