编写一个Time类,并对“-”和“--”运算符重载,使该类的对象可以互相减 和自减 。 注意:两个重载运算符都不能写成Time的成员函数 主函数: int main() { int h1,m1,s1,h2,m2,s2; cin>>h1>>m1>>s1>>h2>>m2>>s2; Time t1(h1,m1,s1), t2(h2,m2,s2), t; t=t1-t2; t.disp(); t--; t.disp(); (--t)--; t.disp(); (t--).disp(); t.disp(); return 0; } Input 键盘输入两个时间t1、t2的时分秒 Output 输出程序的运行结果 Sample Input 3 45 10 1 50 24 Sample Output 1:54'46" 1:54'45" 1:54'43" 1:54'43" 1:54'42"请用c++语言编写代码使其符合以上要求
时间: 2023-12-24 07:04:14 浏览: 35
以下是代码实现:
```c++
#include<iostream>
using namespace std;
class Time {
public:
Time(int h, int m, int s) : hour(h), minute(m), second(s) {}
Time() : hour(0), minute(0), second(0) {}
// 重载减法运算符
friend Time operator-(const Time &t1, const Time &t2) {
int h = t1.hour - t2.hour;
int m = t1.minute - t2.minute;
int s = t1.second - t2.second;
if (s < 0) {
m--;
s += 60;
}
if (m < 0) {
h--;
m += 60;
}
return Time(h, m, s);
}
// 重载前置自减运算符
friend Time &operator--(Time &t) {
t.second--;
if (t.second < 0) {
t.minute--;
t.second += 60;
}
if (t.minute < 0) {
t.hour--;
t.minute += 60;
}
return t;
}
// 重载后置自减运算符
friend Time operator--(Time &t, int) {
Time temp = t;
--t;
return temp;
}
void disp() const {
cout << hour << ":" << minute << "'" << second << "\"" << endl;
}
private:
int hour;
int minute;
int second;
};
int main() {
int h1, m1, s1, h2, m2, s2;
cin >> h1 >> m1 >> s1 >> h2 >> m2 >> s2;
Time t1(h1, m1, s1), t2(h2, m2, s2), t;
t = t1 - t2;
t.disp();
--t;
t.disp();
(--t)--;
t.disp();
(t--).disp();
t.disp();
return 0;
}
```
主要实现思路:
1. 定义 `Time` 类,包括构造函数和 `hour`、`minute`、`second` 三个成员变量。
2. 重载减法运算符 `-`,使得两个 `Time` 类型的对象可以相减,并返回一个新的 `Time` 对象。
3. 重载前置自减运算符 `--`,使得 `Time` 类型的对象可以自减。
4. 重载后置自减运算符 `--`,使得 `Time` 类型的对象可以先输出,再自减。
5. 实现 `disp()` 函数,用于输出时间。
6. 在 `main()` 函数中,依次输入两个时间,创建 `Time` 对象。然后分别进行减法运算、自减运算和自减运算后输出,最后输出原对象的值。
相关推荐
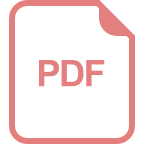















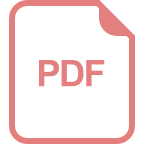