怎样用vue3实现强制横屏或强制竖屏
时间: 2023-07-12 13:10:07 浏览: 211
要实现强制横屏或强制竖屏,可以使用 Vue 3 Composition API 提供的 `watchEffect` 和 `onMounted` 等方法来监听屏幕方向变化,并通过修改样式来实现强制横屏或强制竖屏。
以下是一个简单的示例代码,供参考:
```html
<template>
<div class="fullscreen">
<div class="rotate-info" v-if="showRotateInfo">{{rotateInfo}}</div>
<div class="content">
<!-- your content here -->
</div>
</div>
</template>
<script>
import { ref, watchEffect, onMounted } from 'vue';
export default {
setup() {
const showRotateInfo = ref(false);
const rotateInfo = ref('');
// 监听屏幕方向变化
watchEffect(() => {
const orientation = window.orientation;
if (orientation === 90 || orientation === -90) {
// 横屏
showRotateInfo.value = true;
rotateInfo.value = '请将手机竖过来';
} else if (orientation === 0 || orientation === 180) {
// 竖屏
showRotateInfo.value = true;
rotateInfo.value = '请将手机横过来';
}
});
// 强制横屏或强制竖屏
onMounted(() => {
const orientation = window.orientation;
if (orientation === 90 || orientation === -90) {
// 横屏
document.documentElement.style.transform = 'rotate(-90deg)';
document.documentElement.style.width = '100vh';
document.documentElement.style.height = '100vw';
document.documentElement.style.overflow = 'hidden';
} else if (orientation === 0 || orientation === 180) {
// 竖屏
document.documentElement.style.transform = 'rotate(0deg)';
document.documentElement.style.width = '100vw';
document.documentElement.style.height = '100vh';
document.documentElement.style.overflow = 'hidden';
}
});
return {
showRotateInfo,
rotateInfo
};
}
};
</script>
<style>
.fullscreen {
width: 100%;
height: 100%;
position: fixed;
top: 0;
left: 0;
}
.rotate-info {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
color: #fff;
background-color: rgba(0, 0, 0, 0.8);
position: absolute;
top: 0;
left: 0;
z-index: 999;
}
.content {
width: 100%;
height: 100%;
padding: 20px;
box-sizing: border-box;
}
</style>
```
在上面的示例代码中,我们通过监听 `window.orientation` 属性来判断屏幕方向,然后根据屏幕方向来显示提示信息和修改样式。在 `onMounted` 钩子函数中,我们通过修改 `transform`、`width`、`height` 和 `overflow` 等样式来实现强制横屏或强制竖屏。
注意,由于不同浏览器的实现方式可能不同,上述代码的兼容性可能存在问题,需要在具体情况下进行测试和调整。
相关推荐
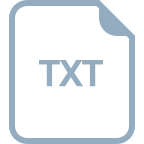
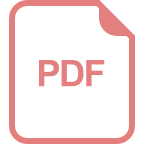














