用pyqt5 将卷积神经网络训练过程实时显示到GUI的Textbrowser上
时间: 2023-07-16 21:14:38 浏览: 61
可以使用PyQt5中的QThread和信号与槽机制来实现将卷积神经网络训练过程实时显示到GUI的Textbrowser上。
首先,我们需要创建一个QThread子类来处理训练过程。在这个子类中,我们可以重写QThread的run方法来执行训练操作。我们还需要定义一个信号,当训练过程中有新的输出时,该信号会被发射。
```python
from PyQt5.QtCore import QThread, pyqtSignal
class TrainThread(QThread):
output_signal = pyqtSignal(str)
def __init__(self, model, train_data, batch_size, epochs):
super().__init__()
self.model = model
self.train_data = train_data
self.batch_size = batch_size
self.epochs = epochs
def run(self):
for epoch in range(self.epochs):
# train the model and get the output
output = "Epoch {} completed".format(epoch)
self.output_signal.emit(output)
```
接下来,在GUI的主窗口中,我们需要创建一个Textbrowser控件来显示训练过程中的输出。我们还需要连接TrainThread的信号到槽函数,这个槽函数将会在信号被发射时被调用,从而更新Textbrowser的内容。
```python
from PyQt5.QtWidgets import QMainWindow, QTextBrowser
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# create the Textbrowser widget
self.text_browser = QTextBrowser(self)
self.setCentralWidget(self.text_browser)
# create the TrainThread object and connect its signal to the slot function
self.train_thread = TrainThread(model, train_data, batch_size, epochs)
self.train_thread.output_signal.connect(self.update_text_browser)
# start the train thread
self.train_thread.start()
def update_text_browser(self, output):
# update the Textbrowser widget with the output
self.text_browser.append(output)
```
现在,当我们启动GUI应用程序时,TrainThread将会被创建并启动。当训练过程中有新的输出时,TrainThread将会发射output_signal信号,该信号将会被连接到update_text_browser槽函数。在这个槽函数中,Textbrowser控件将会被更新。
相关推荐
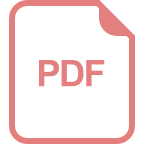
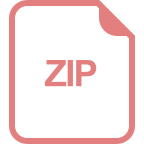
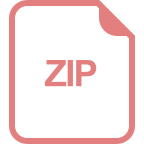
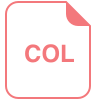
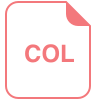
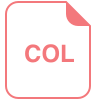
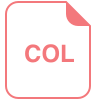
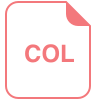









