使用c语言编写程序:data01.dat存放的是一系列整型数据,求data01.dat中的最大十个数的和的立方根(先求十个数的和再求立方根),求得的结果显示在屏幕上,并且将最大的十个数与所求得的结果输出到result.dat中。提示:先对data01.dat中的数据进行排序,然后再进行计算。要求: 1)使用函数double intSumMax(int *p,int num) { } 来实现,并把该函数放在头文件ISmax.h中以便在主函数中调用该函数. 2)主函数中使用的数组使用动态数组来创建. 3)result.dat在程序的执行过程中创建。
时间: 2023-12-10 13:38:13 浏览: 101
首先,我们需要创建头文件 ISmax.h,其中包含函数 double intSumMax(int *p,int num) 的声明:
```c
#ifndef ISMAX_H
#define ISMAX_H
double intSumMax(int *p, int num);
#endif
```
然后,我们创建一个名为 main.c 的文件,其中包含主函数和需要实现的 intSumMax 函数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include "ISmax.h"
int main() {
FILE *fp;
int num = 0, i = 0;
int *arr;
double sumMaxCubeRoot;
// 打开文件
if ((fp = fopen("data01.dat", "rb")) == NULL) {
printf("Cannot open file data01.dat\n");
exit(1);
}
// 获取文件中数据的数量
fseek(fp, 0L, SEEK_END); // 文件指针移动到文件末尾
num = ftell(fp) / sizeof(int); // 获取文件中数据的数量
rewind(fp); // 文件指针移动到文件开头
// 创建动态数组
arr = (int *) malloc(num * sizeof(int));
if (arr == NULL) {
printf("Memory allocation failed!\n");
exit(1);
}
// 从文件中读取数据
fread(arr, sizeof(int), num, fp);
// 关闭文件
fclose(fp);
// 对数组进行排序
qsort(arr, num, sizeof(int), cmp); // cmp 是用来比较两个整数大小的函数
// 计算最大的十个数的和的立方根
sumMaxCubeRoot = intSumMax(arr, num);
sumMaxCubeRoot = cbrt(sumMaxCubeRoot);
// 输出结果
printf("The cube root of the sum of the top 10 maximum numbers is: %lf\n", sumMaxCubeRoot);
// 将最大的十个数和结果写入文件 result.dat
writeResultToFile(arr, sumMaxCubeRoot);
// 释放动态数组
free(arr);
return 0;
}
// 用来比较两个整数大小的函数
int cmp(const void *a, const void *b) {
return (*(int *) b - *(int *) a);
}
// 计算最大的十个数的和,并返回结果
double intSumMax(int *p, int num) {
int i;
double sum = 0;
for (i = 0; i < 10 && i < num; i++) {
sum += p[i];
}
return sum;
}
// 将最大的十个数和结果写入文件 result.dat
void writeResultToFile(int *p, double result) {
FILE *fp;
int i;
// 打开文件
if ((fp = fopen("result.dat", "w")) == NULL) {
printf("Cannot open file result.dat\n");
exit(1);
}
// 写入数据
fprintf(fp, "The top 10 maximum numbers are:\n");
for (i = 0; i < 10 && p[i] >= 0; i++) {
fprintf(fp, "%d ", p[i]);
}
fprintf(fp, "\n");
fprintf(fp, "The cube root of the sum of the top 10 maximum numbers is: %lf\n", result);
// 关闭文件
fclose(fp);
}
```
阅读全文
相关推荐
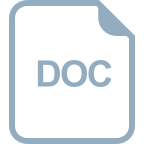
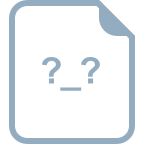


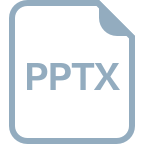
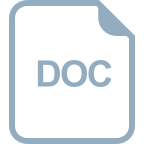
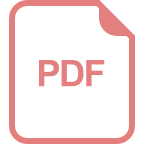
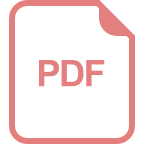
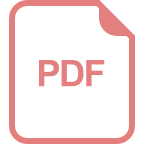
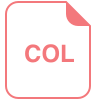

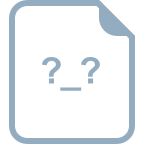
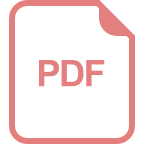
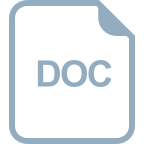
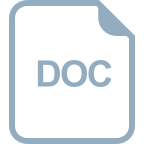
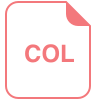
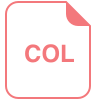