使用vue3实现流程引擎图的界面显示
时间: 2024-05-08 19:17:09 浏览: 220
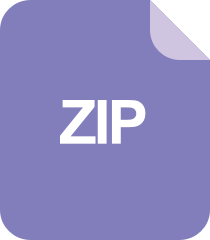
可视化流程引擎RoadFlow v3.0 Vue版
Vue3是一种用于构建用户界面的现代JavaScript框架。流程引擎图则是一种用于表示业务流程的图形化工具。下面是使用Vue3实现流程引擎图的界面显示的步骤:
1. 安装Vue3:首先需要安装Vue3。可以使用npm或yarn进行安装。示例代码如下:
```bash
npm install vue@next
# or
yarn add vue@next
```
2. 安装流程引擎图工具:选择一个流程引擎图工具,比如Flowchart.js。可以使用npm或yarn进行安装。示例代码如下:
```bash
npm install flowchart.js
# or
yarn add flowchart.js
```
3. 创建Vue组件:创建一个Vue组件,用于显示流程引擎图。示例代码如下:
```javascript
<template>
<div>
<div ref="diagram"></div>
</div>
</template>
<script>
import flowchart from 'flowchart.js';
export default {
name: 'FlowchartDiagram',
props: {
chart: {
type: String,
required: true
},
options: {
type: Object,
default: () => ({})
}
},
mounted() {
// 创建流程引擎图
const diagram = flowchart.parse(this.chart);
// 设置选项
Object.assign(diagram, this.options);
// 渲染流程引擎图
diagram.drawSVG(this.$refs.diagram);
}
};
</script>
```
4. 使用Vue组件:将Vue组件添加到Vue应用中,以显示流程引擎图。示例代码如下:
```javascript
<template>
<div>
<FlowchartDiagram :chart="chart" :options="options" />
</div>
</template>
<script>
import FlowchartDiagram from './FlowchartDiagram.vue';
export default {
components: {
FlowchartDiagram
},
data() {
return {
chart: `
st=>start: Start
e=>end: End
op1=>operation: Operation 1
op2=>operation: Operation 2
cond=>condition: Condition
st->op1->cond
cond(yes)->op2->e
cond(no)->e
`,
options: {
'flowstate': {
'active': { 'fill': '#1abc9c', 'font-size': 15 },
'done': { 'fill': '#9b59b6', 'font-size': 15 },
'future': { 'fill': '#34495e', 'font-size': 15 }
},
'end-style': { 'fill': '#e74c3c', 'stroke-width': 0 },
'line-width': 3,
'line-length': 50,
'text-margin': 10,
'font-size': 14,
'font-color': 'black',
'element-color': 'black',
'fill': 'white',
'yes-text': 'yes',
'no-text': 'no',
'arrow-end': 'block',
'scale': 1,
'symbols': {
'start': { 'font-color': 'red', 'element-color': 'green', 'fill': 'yellow' },
'end': { 'class': 'end-element' }
}
}
};
}
};
</script>
```
这样,就可以通过Vue组件显示流程引擎图了。可以根据实际需求调整选项,以满足特定的业务需求。
阅读全文
相关推荐
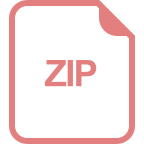
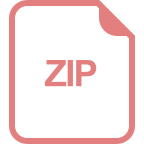
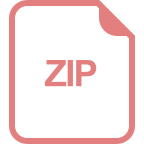
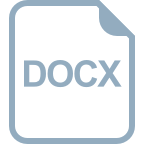
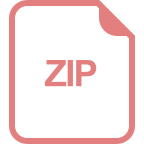
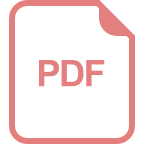
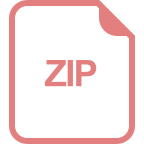
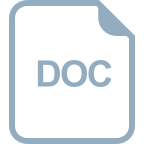
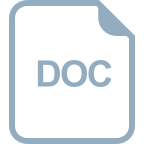
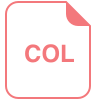
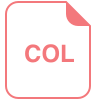
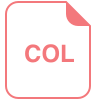
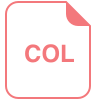
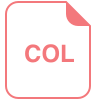
