用matlab创建一个多层CNN,实现二维特征的分类识别
时间: 2024-03-13 12:19:47 浏览: 20
以下是一个使用matlab创建多层CNN进行二维特征分类的示例代码:
首先,需要导入数据集,这里使用的是MatConvNet示例中的cifar-10数据集:
```matlab
% load cifar-10 dataset
untar('http://www.cs.toronto.edu/~kriz/cifar-10-matlab.tar.gz', 'cifar-10-batches-mat');
addpath('cifar-10-batches-mat');
```
接着,定义CNN的结构,包括多个卷积层、池化层和全连接层:
```matlab
% define CNN structure
net.layers = {} ;
% layer 1
net.layers{end+1} = struct('type', 'conv', ...
'weights', {{0.01*randn(5,5,3,32, 'single'), zeros(1, 32, 'single')}}, ...
'stride', 1, ...
'pad', 2) ;
net.layers{end+1} = struct('type', 'relu') ;
net.layers{end+1} = struct('type', 'pool', ...
'method', 'max', ...
'pool', [3 3], ...
'stride', 2, ...
'pad', [0 1 0 1]) ;
% layer 2
net.layers{end+1} = struct('type', 'conv', ...
'weights', {{0.01*randn(5,5,32,64, 'single'), zeros(1,64,'single')}}, ...
'stride', 1, ...
'pad', 2) ;
net.layers{end+1} = struct('type', 'relu') ;
net.layers{end+1} = struct('type', 'pool', ...
'method', 'max', ...
'pool', [3 3], ...
'stride', 2, ...
'pad', [0 1 0 1]) ;
% layer 3
net.layers{end+1} = struct('type', 'conv', ...
'weights', {{0.01*randn(5,5,64,64, 'single'), zeros(1,64,'single')}}, ...
'stride', 1, ...
'pad', 2) ;
net.layers{end+1} = struct('type', 'relu') ;
net.layers{end+1} = struct('type', 'pool', ...
'method', 'max', ...
'pool', [3 3], ...
'stride', 2, ...
'pad', [0 1 0 1]) ;
% layer 4
net.layers{end+1} = struct('type', 'conv', ...
'weights', {{0.01*randn(5,5,64,10, 'single'), zeros(1,10,'single')}}, ...
'stride', 1, ...
'pad', 2) ;
net.layers{end+1} = struct('type', 'softmaxloss') ;
```
然后,使用cnn_train函数进行训练:
```matlab
% train CNN
opts.train.batchSize = 100 ;
opts.train.numEpochs = 20 ;
opts.train.continue = true ;
opts.train.gpus = [] ;
opts.train.learningRate = 0.001 ;
opts.train.momentum = 0.9 ;
opts.train.weightDecay = 0.0005 ;
% prepare data
train = load('data_batch_1.mat');
train_data = single(permute(reshape(train.data',32,32,3,[]),[2 1 3 4]));
train_labels = single(train.labels'+1);
val = load('data_batch_2.mat');
val_data = single(permute(reshape(val.data',32,32,3,[]),[2 1 3 4]));
val_labels = single(val.labels'+1);
% train CNN
cnn = cnn_train(net, train_data, train_labels, val_data, val_labels, opts);
```
最后,使用cnn_test函数进行测试:
```matlab
% test CNN
test = load('test_batch.mat') ;
test_data = single(permute(reshape(test.data',32,32,3,[]),[2 1 3 4]));
test_labels = single(test.labels'+1);
% classify test images
scores = cnn_test(cnn, test_data) ;
% compute accuracy
[~, predictions] = max(scores) ;
acc = mean(predictions == test_labels) ;
fprintf('Accuracy: %f\n', acc) ;
```
这个示例代码中,使用了三个卷积层和三个池化层,以及一个全连接层和一个softmax层,最终实现了对cifar-10数据集中的图像进行分类识别。具体的CNN结构可以根据需要进行修改和调整。
相关推荐
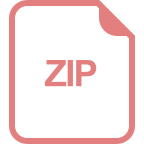
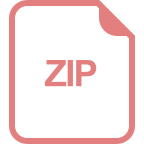
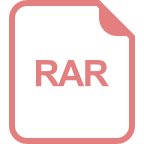














