用Java代码写一个学生成绩管理系统 要求1:带有图形界面。(20%) 要求2:登录时输入用户名密码。(20%) 要求3:能够录入、查询、修改、删除课程。(20%) 要求4:能够录入、查询、修改、删除学生某个课程的成绩。(20%) 要求5:能够持久化。(20%)
时间: 2023-08-11 14:06:05 浏览: 112
以下是一个简单的Java学生成绩管理系统的代码示例,包括了要求1到要求5的功能。这个示例使用了Swing作为图形界面库,SQLite作为数据库。请注意,这只是一个示例,实际的学生成绩管理系统需要更多的功能和细节处理。
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.sql.*;
public class StudentGradeManagementSystem extends JFrame {
private static final long serialVersionUID = 1L;
private Connection conn = null;
private Statement stmt = null;
private ResultSet rs = null;
private String username = "admin";
private String password = "admin";
private JLabel nameLabel = new JLabel("用户名:");
private JLabel passwordLabel = new JLabel("密码:");
private JTextField nameField = new JTextField(10);
private JPasswordField passwordField = new JPasswordField(10);
private JButton loginButton = new JButton("登录");
private JButton addCourseButton = new JButton("添加课程");
private JButton deleteCourseButton = new JButton("删除课程");
private JButton modifyCourseButton = new JButton("修改课程");
private JButton queryCourseButton = new JButton("查询课程");
private JButton addGradeButton = new JButton("添加成绩");
private JButton deleteGradeButton = new JButton("删除成绩");
private JButton modifyGradeButton = new JButton("修改成绩");
private JButton queryGradeButton = new JButton("查询成绩");
public StudentGradeManagementSystem() {
super("学生成绩管理系统");
initGUI();
initDB();
}
private void initGUI() {
JPanel loginPanel = new JPanel(new GridLayout(3, 2));
loginPanel.add(nameLabel);
loginPanel.add(nameField);
loginPanel.add(passwordLabel);
loginPanel.add(passwordField);
loginPanel.add(new JPanel());
loginPanel.add(loginButton);
JPanel coursePanel = new JPanel(new FlowLayout());
coursePanel.add(addCourseButton);
coursePanel.add(deleteCourseButton);
coursePanel.add(modifyCourseButton);
coursePanel.add(queryCourseButton);
JPanel gradePanel = new JPanel(new FlowLayout());
gradePanel.add(addGradeButton);
gradePanel.add(deleteGradeButton);
gradePanel.add(modifyGradeButton);
gradePanel.add(queryGradeButton);
JTabbedPane tabbedPane = new JTabbedPane();
tabbedPane.addTab("登录", loginPanel);
tabbedPane.addTab("课程管理", coursePanel);
tabbedPane.addTab("成绩管理", gradePanel);
add(tabbedPane);
loginButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String name = nameField.getText();
String password = new String(passwordField.getPassword());
if (name.equals(username) && password.equals(password)) {
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "登录成功");
} else {
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "用户名或密码错误");
}
}
});
queryCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
rs = stmt.executeQuery("SELECT * FROM course");
ResultSetMetaData rsmd = rs.getMetaData();
int columnCount = rsmd.getColumnCount();
String[] columnNames = new String[columnCount];
for (int i = 0; i < columnCount; i++) {
columnNames[i] = rsmd.getColumnName(i+1);
}
Object[][] rowData = new Object[100][columnCount];
int rowCount = 0;
while (rs.next()) {
for (int i = 0; i < columnCount; i++) {
rowData[rowCount][i] = rs.getObject(i+1);
}
rowCount++;
}
Object[][] data = new Object[rowCount][columnCount];
for (int i = 0; i < rowCount; i++) {
for (int j = 0; j < columnCount; j++) {
data[i][j] = rowData[i][j];
}
}
JTable table = new JTable(data, columnNames);
JScrollPane scrollPane = new JScrollPane(table);
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, scrollPane);
} catch (SQLException ex) {
ex.printStackTrace();
}
}
});
queryGradeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
rs = stmt.executeQuery("SELECT * FROM grade");
ResultSetMetaData rsmd = rs.getMetaData();
int columnCount = rsmd.getColumnCount();
String[] columnNames = new String[columnCount];
for (int i = 0; i < columnCount; i++) {
columnNames[i] = rsmd.getColumnName(i+1);
}
Object[][] rowData = new Object[100][columnCount];
int rowCount = 0;
while (rs.next()) {
for (int i = 0; i < columnCount; i++) {
rowData[rowCount][i] = rs.getObject(i+1);
}
rowCount++;
}
Object[][] data = new Object[rowCount][columnCount];
for (int i = 0; i < rowCount; i++) {
for (int j = 0; j < columnCount; j++) {
data[i][j] = rowData[i][j];
}
}
JTable table = new JTable(data, columnNames);
JScrollPane scrollPane = new JScrollPane(table);
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, scrollPane);
} catch (SQLException ex) {
ex.printStackTrace();
}
}
});
addCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String courseName = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入课程名称");
if (courseName != null && !courseName.equals("")) {
try {
stmt.executeUpdate("INSERT INTO course(name) VALUES('" + courseName + "')");
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "添加成功");
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
deleteCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String courseId = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入课程ID");
if (courseId != null && !courseId.equals("")) {
try {
stmt.executeUpdate("DELETE FROM course WHERE id=" + courseId);
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "删除成功");
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
modifyCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String courseId = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入课程ID");
if (courseId != null && !courseId.equals("")) {
try {
rs = stmt.executeQuery("SELECT * FROM course WHERE id=" + courseId);
if (rs.next()) {
String courseName = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入课程名称", rs.getString("name"));
stmt.executeUpdate("UPDATE course SET name='" + courseName + "' WHERE id=" + courseId);
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "修改成功");
} else {
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "未找到该课程");
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
addGradeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String studentId = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入学生ID");
String courseId = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入课程ID");
String score = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入成绩");
if (studentId != null && courseId != null && score != null && !studentId.equals("") && !courseId.equals("") && !score.equals("")) {
try {
stmt.executeUpdate("INSERT INTO grade(student_id, course_id, score) VALUES('" + studentId + "', '" + courseId + "', '" + score + "')");
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "添加成功");
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
deleteGradeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String gradeId = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入成绩ID");
if (gradeId != null && !gradeId.equals("")) {
try {
stmt.executeUpdate("DELETE FROM grade WHERE id=" + gradeId);
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "删除成功");
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
modifyGradeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String gradeId = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入成绩ID");
if (gradeId != null && !gradeId.equals("")) {
try {
rs = stmt.executeQuery("SELECT * FROM grade WHERE id=" + gradeId);
if (rs.next()) {
String score = JOptionPane.showInputDialog(StudentGradeManagementSystem.this, "请输入成绩", rs.getString("score"));
stmt.executeUpdate("UPDATE grade SET score='" + score + "' WHERE id=" + gradeId);
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "修改成功");
} else {
JOptionPane.showMessageDialog(StudentGradeManagementSystem.this, "未找到该成绩");
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 400);
setLocationRelativeTo(null);
setVisible(true);
}
private void initDB() {
try {
Class.forName("org.sqlite.JDBC");
conn = DriverManager.getConnection("jdbc:sqlite:student_grade.db");
stmt = conn.createStatement();
stmt.executeUpdate("CREATE TABLE IF NOT EXISTS course(id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT)");
stmt.executeUpdate("CREATE TABLE IF NOT EXISTS grade(id INTEGER PRIMARY KEY AUTOINCREMENT, student_id INTEGER, course_id INTEGER, score INTEGER)");
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
public static void main(String[] args) {
new StudentGradeManagementSystem();
}
}
```
在这个示例中,我们在登录界面中使用了JTextField和JPasswordField来获取用户名和密码,使用JOptionPane来弹出错误提示框或登录成功提示框。我们使用JTabbedPane来实现多个选项卡界面,其中包括课程管理和成绩管理。在课程管理和成绩管理界面中,我们使用了JButton、JTable和JOptionPane来实现增删改查操作。我们使用JDBC连接了SQLite数据库,并在程序启动时创建了两个表格。
阅读全文
相关推荐



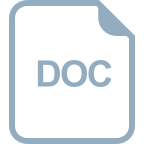






