能否为我提供一个详细的基于JAVA实现贪吃蛇游戏的代码
时间: 2024-09-12 16:06:34 浏览: 34
编写一个完整的贪吃蛇游戏涉及到多个部分,包括游戏逻辑、图形用户界面(GUI)、事件处理等。在Java中,我们通常使用Swing库来创建GUI。以下是一个简化版的贪吃蛇游戏的基本框架代码,用于演示如何开始构建这样一个游戏。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.util.ArrayList;
import java.util.Random;
public class SnakeGame extends JPanel implements ActionListener {
private final int WIDTH = 300;
private final int HEIGHT = 300;
private final int DOT_SIZE = 10; // 蛇身体的大小
private final int ALL_DOTS = 900; // 游戏区域能容纳的最大点数
private final int RANDOM_POSITION = 29; // 用于计算食物随机位置的数字
private final int x[] = new int[ALL_DOTS]; // 蛇x坐标数组
private final int y[] = new int[ALL_DOTS]; // 蛇y坐标数组
private int bodyParts;
private int applesEaten;
private int appleX; // 苹果的X坐标
private int appleY; // 苹果的Y坐标
private char direction = 'R'; // 初始方向向右
private boolean running = false;
private Timer timer;
private Random random;
public SnakeGame() {
initGame();
}
private void initGame() {
addKeyListener(new TAdapter());
setBackground(Color.black);
setFocusable(true);
setPreferredSize(new Dimension(WIDTH, HEIGHT));
startGame();
}
private void startGame() {
bodyParts = 3; // 初始蛇的长度
applesEaten = 0;
for (int i = 0; i < bodyParts; i++) {
x[i] = 50 - i * DOT_SIZE;
y[i] = 50;
}
locateApple();
running = true;
timer = new Timer(140, this);
timer.start();
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
draw(g);
}
private void draw(Graphics g) {
if (running) {
g.setColor(Color.red);
g.fillOval(appleX, appleY, DOT_SIZE, DOT_SIZE);
for (int i = 0; i < bodyParts; i++) {
if (i == 0) {
g.setColor(Color.green);
g.fillRect(x[i], y[i], DOT_SIZE, DOT_SIZE);
} else {
g.setColor(new Color(45, 180, 0));
g.fillRect(x[i], y[i], DOT_SIZE, DOT_SIZE);
}
}
} else {
gameOver(g);
}
}
private void gameOver(Graphics g) {
// 显示游戏结束文本
g.setColor(Color.red);
g.setFont(new Font("Arial", Font.BOLD, 40));
FontMetrics metrics = getFontMetrics(g.getFont());
g.drawString("Game Over", (WIDTH - metrics.stringWidth("Game Over")) / 2, HEIGHT / 2);
}
private void checkApple() {
// 检查蛇是否吃到苹果
}
private void move() {
// 移动蛇的身体
}
private void checkCollision() {
// 检查蛇是否碰到自己或墙壁
}
private void locateApple() {
// 随机放置苹果
}
@Override
public void actionPerformed(ActionEvent e) {
if (running) {
checkApple();
checkCollision();
move();
}
repaint();
}
private class TAdapter extends KeyAdapter {
@Override
public void keyPressed(KeyEvent e) {
// 响应按键,改变蛇的方向
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new SnakeGame());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
});
}
}
```
这段代码只是一个起点,它定义了游戏窗口和一些基本变量。其中`move()`, `checkApple()`, `checkCollision()`, `locateApple()`, 和键盘事件处理方法(`keyPressed()`)需要你自己去实现。这个框架已经设置好了游戏循环和画布更新机制。还需要编写相应的逻辑来处理蛇的移动、苹果的生成、碰撞检测等核心游戏功能。
由于篇幅和复杂性的原因,上述代码并没有完整实现贪吃蛇游戏的所有功能。完整的游戏需要进一步编写逻辑来完成移动、增长、食物生成和碰撞检测等。
阅读全文
相关推荐
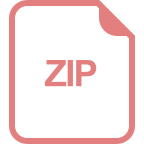
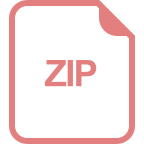
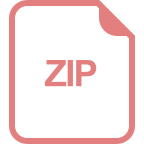
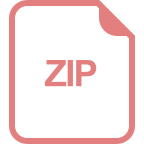
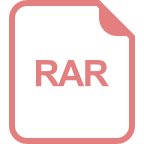
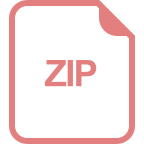
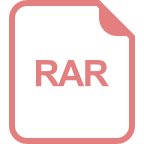
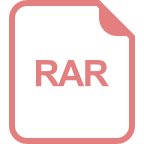
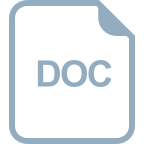
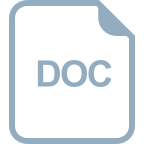
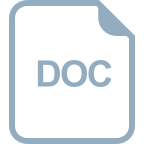
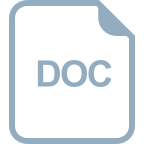
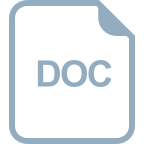
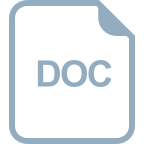
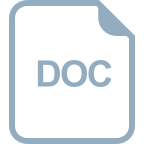


