ibo递归方法: 定义一个求Fibonacci(斐波那契)数列的函数 fb(n),输出前20项。每项宽度5字符,右对 齐,每行输出10个。 def fib(n): if n<=(1)_:return(1) else: return((2). n=20 for i in range(1,n+1): print(formatl(fb(i),"_(3)_",end=')#宽
时间: 2023-12-12 22:02:27 浏览: 95
好的,这段代码有一些问题,我来说明一下:
```python
def fib(n):
if n <= 1:
return n
else:
return fib(n-1) + fib(n-2)
n = 20
for i in range(1, n+1):
print(format(fib(i), ">5"), end='')
if i % 10 == 0:
print()
```
需要注意的是:
1. 在递归函数中,当n<=1时,应该返回n,而不是1。
2. 在递归函数中,应该返回fib(n-1)和fib(n-2)的和,而不是(n-1)和(n-2)。
3. 在print语句中,可以使用format函数来格式化输出,">5"表示右对齐,宽度为5个字符。
4. 在print语句中,每输出10个数就换行,可以使用i % 10 == 0来判断。
阅读全文
相关推荐
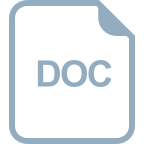
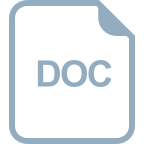
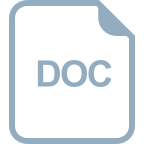
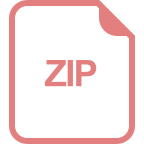
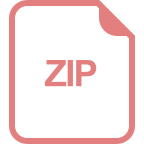
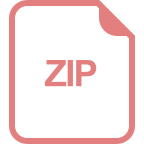
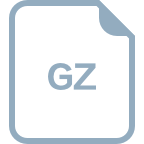
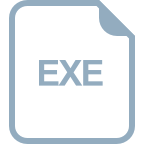
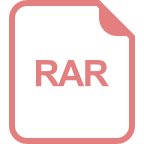
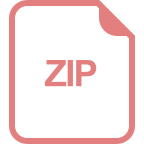
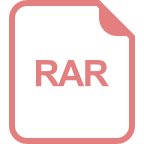
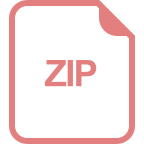
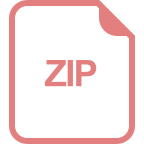
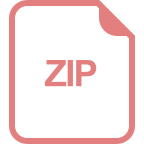
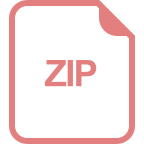
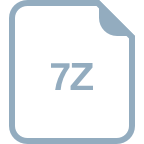