inc ebx; cmp ebx,dword[ebp+12] jne cycle; mov eax,esi; pop esi; pop ebx; pop edi; leave ret这段代码在nasm中的作用
时间: 2024-05-26 07:12:26 浏览: 131
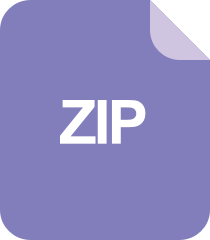
32位 80x86 汇编语言基础(课件+附件software)
这段代码是汇编语言的代码,它的作用是实现循环计数器的功能,通过逐个增加ebx寄存器的值,判断是否达到循环结束条件,来控制流程的执行。当ebx的值不等于dword[ebp+12]时,跳转到cycle标签处继续执行。如果ebx的值等于dword[ebp+12],则跳出循环,执行mov、pop、leave和ret等指令,返回程序调用处。
阅读全文
相关推荐
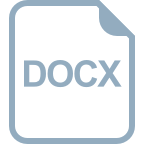
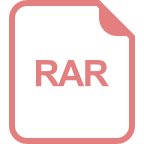
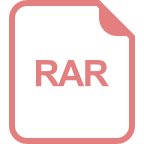



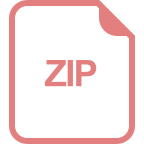
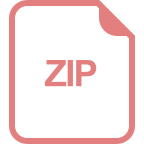