android在onCreateDialog中使用AlertDialog 加载设置自定义一个弹窗布局完整xml与java代码并实现弹窗透明背景
时间: 2023-12-10 10:37:23 浏览: 223
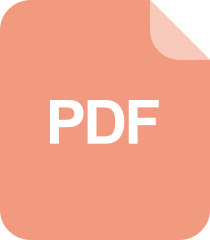
Android编程实现对话框Dialog背景透明功能示例
以下是一个在 `onCreateDialog` 方法中使用 `AlertDialog` 并实现透明背景的例子:
1. 首先在 res/layout 目录下创建一个自定义的弹窗布局文件,例如 `dialog_custom.xml`:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/dialog_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/dialog_background"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/dialog_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="标题"
android:textSize="18sp" />
<EditText
android:id="@+id/dialog_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入内容"
android:inputType="text" />
<Button
android:id="@+id/dialog_confirm"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="确定" />
<Button
android:id="@+id/dialog_cancel"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="取消" />
</LinearLayout>
```
2. 在 res/drawable 目录下创建一个透明背景的 drawable 文件,例如 `dialog_background.xml`:
```xml
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="#00000000" />
<corners android:radius="8dp" />
</shape>
```
3. 在 Activity 中实现 `onCreateDialog` 方法,加载自定义的弹窗布局文件并设置透明背景:
```java
@Override
protected Dialog onCreateDialog(int id) {
if (id == DIALOG_CUSTOM) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
LayoutInflater inflater = LayoutInflater.from(this);
View view = inflater.inflate(R.layout.dialog_custom, null);
builder.setView(view);
AlertDialog dialog = builder.create();
dialog.getWindow().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
// 设置按钮点击事件
Button confirmBtn = view.findViewById(R.id.dialog_confirm);
Button cancelBtn = view.findViewById(R.id.dialog_cancel);
confirmBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 确定按钮点击事件
dialog.dismiss();
}
});
cancelBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 取消按钮点击事件
dialog.dismiss();
}
});
return dialog;
}
return super.onCreateDialog(id);
}
```
以上代码中,我们首先创建一个 `AlertDialog.Builder` 对象,并使用 `LayoutInflater` 加载自定义布局文件 `dialog_custom.xml`。然后将布局文件设置为对话框的视图,并创建 AlertDialog 对象。接着,我们使用 `getWindow()` 方法获取对话框的窗口对象,并使用 `setBackgroundDrawable()` 方法将窗口背景设置为透明色。最后,我们为确定按钮和取消按钮设置点击事件,并返回创建的 AlertDialog 对象。
在需要弹出对话框的时候,可以使用 `showDialog(DIALOG_CUSTOM)` 方法来显示自定义对话框。
阅读全文
相关推荐
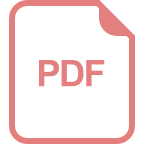
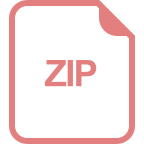




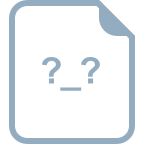
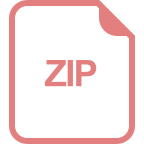
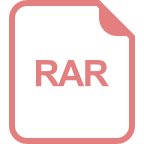
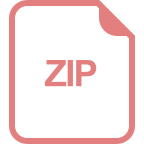
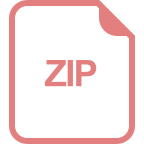
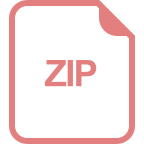
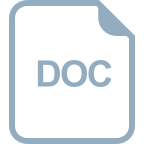
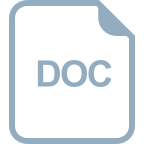
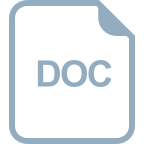


