如何实现 promise.map,限制 promise 并发数
时间: 2023-07-17 09:58:07 浏览: 78
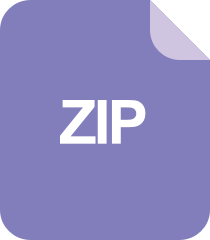
js代码-利用promise 手写一个限制最大并发的类
要实现 `promise.map` 并限制并发数,可以使用 `Promise`、`async/await` 和 `setTimeout` 来进行控制。以下是一个示例实现:
```javascript
function promiseMap(arr, mapper, concurrency) {
return new Promise((resolve, reject) => {
const results = [];
let index = 0;
let running = 0;
let completed = 0;
function run() {
if (completed === arr.length) {
resolve(results);
return;
}
while (running < concurrency && index < arr.length) {
const currentItem = arr[index];
const currentIndex = index;
index++;
running++;
mapper(currentItem)
.then((result) => {
results[currentIndex] = result;
})
.catch((error) => {
reject(error);
})
.finally(() => {
running--;
completed++;
run();
});
}
}
run();
});
}
```
此实现将 `arr` 数组中的每个元素传递给 `mapper` 函数进行处理,并限制最大同时运行的 `concurrency` 数量。函数返回一个 `Promise`,当所有 promises 执行完成后,将解析为包含结果的数组。
使用示例:
```javascript
const items = [1, 2, 3, 4, 5];
const mapper = (item) => {
return new Promise((resolve) => {
setTimeout(() => {
console.log(`Processing item ${item}`);
resolve(item * 2);
}, Math.random() * 1000);
});
};
promiseMap(items, mapper, 2)
.then((results) => {
console.log('Results:', results);
})
.catch((error) => {
console.error('Error:', error);
});
```
在示例中,`items` 数组中的每个元素将通过 `mapper` 函数进行处理,并限制最大同时运行的并发数为 2。处理完成后,将输出结果数组。
请注意,由于 `setTimeout` 是异步的,输出结果的顺序可能会有所不同。
阅读全文
相关推荐
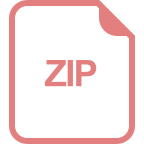
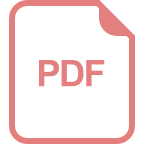
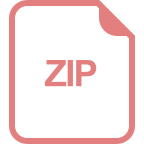
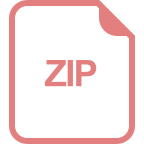
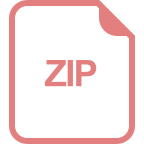
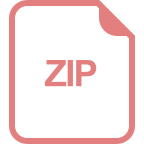
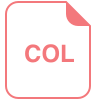
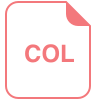
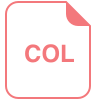
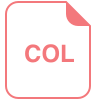
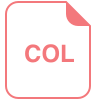
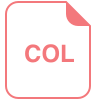
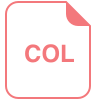
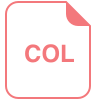
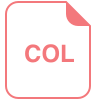
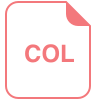
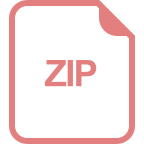