python+pyechart编写数据变动的仪表和直方图
时间: 2024-03-12 22:44:01 浏览: 24
下面是一个使用Python和Pyecharts库编写数据变动的仪表和直方图的示例代码:
```python
from random import randint
from pyecharts import Gauge, Bar
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout
from PyQt5.QtWebEngineWidgets import QWebEngineView
from PyQt5.QtCore import QUrl
# 生成随机数据
value = randint(0, 100)
data = [randint(0, 100) for _ in range(10)]
class GaugeChart(QWidget):
def __init__(self, parent=None):
super(GaugeChart, self).__init__(parent)
self.browser = QWebEngineView()
layout = QVBoxLayout(self)
layout.addWidget(self.browser)
self.chart = Gauge("仪表盘示例", "单位(%)")
self.chart.add("", "", value)
self.chart.render()
self.browser.load(QUrl.fromLocalFile(self.chart._option['renderAsImage'] + ".png"))
class BarChart(QWidget):
def __init__(self, parent=None):
super(BarChart, self).__init__(parent)
self.browser = QWebEngineView()
layout = QVBoxLayout(self)
layout.addWidget(self.browser)
self.chart = Bar("直方图示例", "单位(%)")
self.chart.add("", ["data{}".format(i) for i in range(10)], data)
self.chart.render()
self.browser.load(QUrl.fromLocalFile(self.chart._option['renderAsImage'] + ".png"))
if __name__ == '__main__':
app = QApplication([])
gauge_chart = GaugeChart()
bar_chart = BarChart()
gauge_chart.show()
bar_chart.show()
# 定时器更新数据
timer = QTimer()
timer.timeout.connect(lambda: update_data())
timer.start(1000)
def update_data():
global value, data
value = randint(0, 100)
data = [randint(0, 100) for _ in range(10)]
gauge_chart.chart._option['series'][0]['data'][0]['value'] = value
gauge_chart.chart.render()
gauge_chart.browser.load(QUrl.fromLocalFile(gauge_chart.chart._option['renderAsImage'] + ".png"))
bar_chart.chart._option['series'][0]['data'] = data
bar_chart.chart.render()
bar_chart.browser.load(QUrl.fromLocalFile(bar_chart.chart._option['renderAsImage'] + ".png"))
app.exec_()
```
该示例代码创建了一个仪表盘和一个直方图,并且使用了PyQt5库中的QTimer类定时更新数据。你可以根据自己的需求进行更改和扩展。
相关推荐
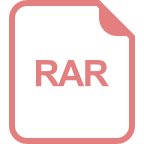
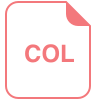
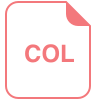
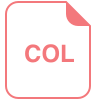











