基于python3的遗传算法优化lightgbm代码
时间: 2023-09-08 19:05:14 浏览: 195
### 回答1:
我们可以使用基于Python3的遗传算法库,如 DEAP,来优化LightGBM代码。 DEAP可以让我们在代码中定义目标函数,并通过遗传算法来计算参数的最优解。
首先,我们需要安装 DEAP 库,可以使用以下命令进行安装:
```
pip install deap
```
然后,我们可以定义目标函数,该函数评估给定的 LightGBM 参数是否是最优的。 可以使用 scikit-learn 等机器学习库来评估模型的性能。
最后,我们可以使用 DEAP 库中的遗传算法,如遗传程序或遗传算法,来对 LightGBM 参数进行优化。
以下是一个简单的示例代码:
```
import numpy as np
from deap import base
from deap import creator
from deap import tools
# Define the evaluation function
def evalFunction(individual):
# Convert the individual to LightGBM parameters
params = {
"max_depth": int(individual[0]),
"num_leaves": int(individual[1]),
"learning_rate": individual[2],
# Add other parameters as needed
}
# Train the LightGBM model with the given parameters
model = train_lightgbm(params)
# Evaluate the performance of the model
score = evaluate_model(model)
return score,
# Define the optimization problem
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_int", np.random.randint, 2, 10)
toolbox.register("attr_float", np.random.uniform, 0.01, 1.0)
toolbox.register("individual", tools.initCycle, creator.Individual,
(toolbox.attr_int, toolbox.attr_int, toolbox.attr_float), n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", evalFunction)
toolbox.register("mate", tools
### 回答2:
基于Python3的遗传算法优化LightGBM代码可以通过以下几个步骤实现:
1. 数据准备:首先,需要准备训练集和测试集数据。可以使用Pandas库读取数据文件,并将数据集分为特征和目标变量。
2. 参数设置:为LightGBM模型设置一些初始参数。可以使用GridSearchCV或RandomizedSearchCV等方法来寻找最佳参数。
3. 创建遗传算法:使用遗传算法库,如DEAP(Distributed Evolutionary Algorithms in Python)或PyGAD(Python Genetic Algorithm Library),来创建遗传算法。设置适应度函数,基因编码等。
4. 评价函数:为了评估每个个体的适应度,可以使用K折交叉验证方法对模型进行训练和验证。将训练集分成k个子集,其中一个子集作为验证集,其余子集作为训练集。通过计算平均验证集的准确率或其他评估指标来评价个体的适应度。
5. 遗传算法的迭代:根据设定的迭代次数或终止条件,运行遗传算法。每一代中,通过选择、交叉和变异等遗传操作对个体进行进化。
6. 模型训练和预测:在每一代中,通过LightGBM模型进行训练,并使用验证集评估模型性能。每次迭代后,选择适应度最高的个体作为最佳模型。
7. 结果分析:分析每次迭代后得到的最佳模型的参数和性能指标,并观察遗传算法的优化过程。根据需要可以调整遗传算法的参数,以达到更理想的结果。
通过以上步骤,可以实现基于Python3的遗传算法优化LightGBM代码。这种方法可以帮助我们找到LightGBM模型的最佳超参数组合,从而提高模型的性能和泛化能力。
阅读全文
相关推荐
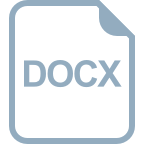
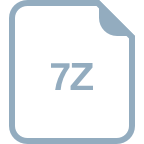
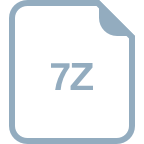
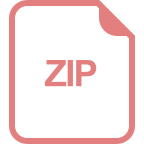
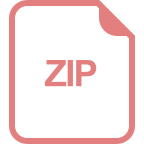
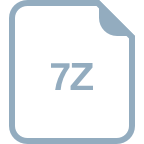
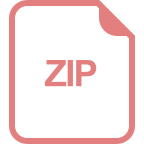
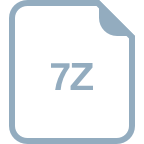
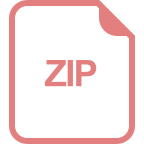
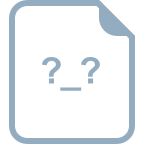