实现一个前后端交互的增删改查
时间: 2023-07-06 13:24:37 浏览: 87
好的,我可以为您提供一些通用的实现方法。首先,您需要确定您的后端 API 的接口文档,包括每个 API 的 URL、请求方式、请求参数和返回结果。然后,在前端,您可以使用 JavaScript 或者其他前端框架(如 React、Vue 等)来发送 HTTP 请求并处理响应,实现增删改查操作。
举个例子,假设您有一个需要管理用户的应用程序,您可以定义以下 API:
- GET /api/users:获取用户列表
- POST /api/users:创建新用户
- GET /api/users/:id:获取指定 ID 的用户
- PUT /api/users/:id:更新指定 ID 的用户
- DELETE /api/users/:id:删除指定 ID 的用户
在前端,您可以使用 fetch 或 axios 等库来发送 HTTP 请求,例如:
```javascript
// 获取用户列表
fetch('/api/users')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
// 创建新用户
fetch('/api/users', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ name: 'John Doe', age: 30 })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
// 获取指定 ID 的用户
fetch('/api/users/123')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
// 更新指定 ID 的用户
fetch('/api/users/123', {
method: 'PUT',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ name: 'Jane Doe', age: 25 })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
// 删除指定 ID 的用户
fetch('/api/users/123', { method: 'DELETE' })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
```
需要注意的是,实现增删改查操作需要对请求和响应进行验证和处理,以确保数据的正确性和完整性。同时,在前端,您还需要根据业务需求展示数据和交互界面,这部分需要根据具体情况进行实现。
阅读全文
相关推荐
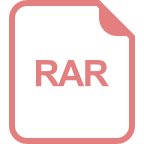
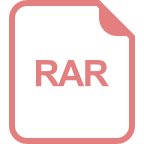
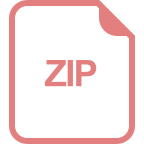
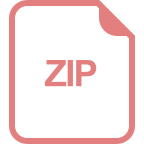
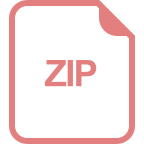
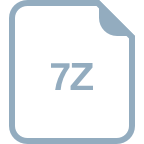
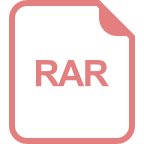
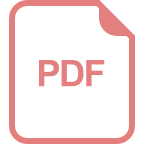
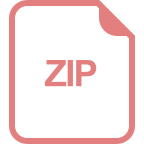
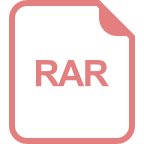
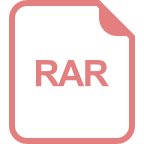
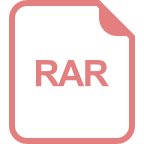
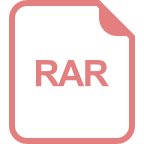
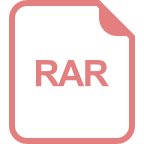
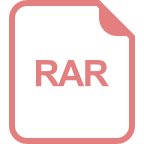
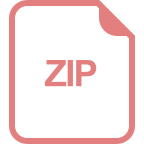
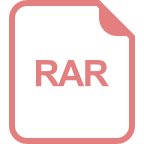
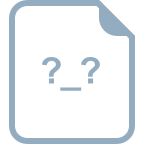