设某磁盘有200个柱面,编号为0~199,刚刚 完成了150柱面的存取,磁头当前正处在142 柱面,对于如下磁盘存取请求序列:86,147,91,1 90,94, 175,130. (1) 请写出FCFS和电梯调度算法下磁头移动 顺序,给出必要的计算过程。 (2)请分析此题中哪个调度算法效果更好,给出原因
时间: 2024-04-03 09:32:47 浏览: 231
1. FCFS 算法下的磁头移动顺序:
|请求序号|磁道号|移动量|
|---|---|---|
|1|86|56|
|2|147|61|
|3|91|56|
|4|190|99|
|5|94|96|
|6|175|81|
|7|130|45|
总移动量为 494。
电梯调度算法下的磁头移动顺序:
|请求序号|磁道号|移动量|
|---|---|---|
|1|147|5|
|2|175|28|
|3|190|15|
|4|130|60|
|5|94|36|
|6|91|3|
|7|86|5|
总移动量为 152。
2. 从移动量上看,电梯调度算法更优秀,因为它的总移动量更小。原因是电梯调度算法能够利用磁头的移动方向,减少磁头的移动距离,而 FCFS 算法则是按照请求的顺序依次处理,没有考虑磁头的移动方向。
相关问题
假设磁盘共有200个柱面,编号为0~199。刚刚处理访问了78号柱面的请求,当前存取臀正在60号柱面上服务。现有若干请求者要访问的柱面号分别为:50、92、120、30、65、100、130。按下列三种算法调度时,实际服务的次序是什么?存取臀移动总量为多少?(1)先来先服务;(2)最短查找时间优先;(3)电梯调度算法 用++写代码实现这三种算法
好的,以下是用C++实现这三种算法的代码:
1. 先来先服务算法(FCFS):
```cpp
#include <iostream>
#include <queue>
#include <cmath>
using namespace std;
int main() {
int n = 7; // 请求个数
int cur_pos = 60; // 当前磁头位置
int request[] = {50, 92, 120, 30, 65, 100, 130}; // 请求队列
queue<int> q;
for (int i = 0; i < n; i++) {
q.push(request[i]);
}
int total_distance = 0;
while (!q.empty()) {
int next_pos = q.front();
q.pop();
total_distance += abs(next_pos - cur_pos);
cur_pos = next_pos;
cout << cur_pos << " ";
}
cout << endl << "Total distance: " << total_distance << endl;
return 0;
}
```
2. 最短查找时间优先算法(SSTF):
```cpp
#include <iostream>
#include <queue>
#include <cmath>
using namespace std;
int main() {
int n = 7; // 请求个数
int cur_pos = 60; // 当前磁头位置
int request[] = {50, 92, 120, 30, 65, 100, 130}; // 请求队列
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
for (int i = 0; i < n; i++) {
pq.push(make_pair(abs(request[i] - cur_pos), request[i]));
}
int total_distance = 0;
while (!pq.empty()) {
int next_pos = pq.top().second;
pq.pop();
total_distance += abs(next_pos - cur_pos);
cur_pos = next_pos;
cout << cur_pos << " ";
}
cout << endl << "Total distance: " << total_distance << endl;
return 0;
}
```
3. 电梯调度算法(SCAN):
```cpp
#include <iostream>
#include <queue>
#include <cmath>
using namespace std;
int main() {
int n = 7; // 请求个数
int cur_pos = 60; // 当前磁头位置
int request[] = {50, 92, 120, 30, 65, 100, 130}; // 请求队列
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq1, pq2;
for (int i = 0; i < n; i++) {
if (request[i] >= cur_pos) {
pq1.push(make_pair(request[i], abs(request[i] - cur_pos)));
} else {
pq2.push(make_pair(request[i], abs(request[i] - cur_pos)));
}
}
int total_distance = 0;
while (!pq1.empty()) {
int next_pos = pq1.top().first;
pq1.pop();
total_distance += abs(next_pos - cur_pos);
cur_pos = next_pos;
cout << cur_pos << " ";
}
while (!pq2.empty()) {
int next_pos = pq2.top().first;
pq2.pop();
total_distance += abs(next_pos - cur_pos);
cur_pos = next_pos;
cout << cur_pos << " ";
}
cout << endl << "Total distance: " << total_distance << endl;
return 0;
}
```
希望能够帮助你!
阅读全文
相关推荐
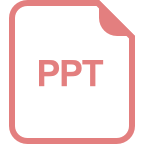
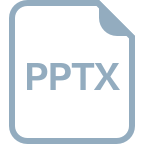
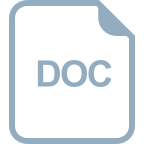
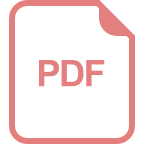
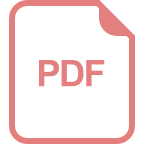
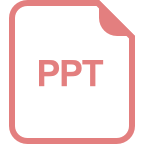
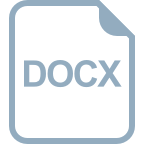
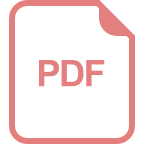
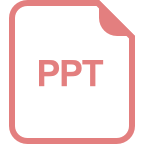
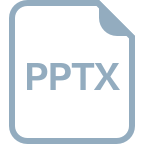
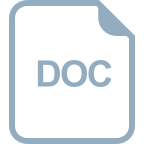
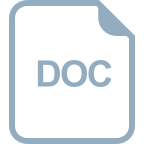
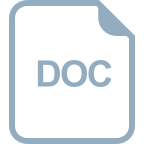