请用C#语言写一个完整程序 动态显示从串口监测的数据,图形显示可以精美一些
时间: 2024-03-19 10:43:33 浏览: 32
以下是一个完整的C#程序,它可以从串口监测数据,并使用OxyPlot库动态展示数据的图形。这个程序使用了WPF框架,因此需要创建一个WPF应用程序,并在MainWindow.xaml中添加一个OxyPlot控件。在MainWindow.xaml.cs文件中添加以下代码:
```csharp
using System;
using System.IO.Ports;
using System.Windows;
using System.Windows.Threading;
using OxyPlot;
using OxyPlot.Series;
namespace SerialPlotter
{
public partial class MainWindow : Window
{
private SerialPort serialPort; // 串口对象
private DispatcherTimer timer; // 定时器
private LineSeries series; // OxyPlot线型图对象
public MainWindow()
{
InitializeComponent();
// 初始化串口对象
serialPort = new SerialPort("COM1", 9600, Parity.None, 8, StopBits.One);
serialPort.DataReceived += SerialPort_DataReceived;
serialPort.Open();
// 初始化OxyPlot图形
var plotModel = new PlotModel();
series = new LineSeries();
plotModel.Series.Add(series);
plot.Model = plotModel;
// 初始化定时器
timer = new DispatcherTimer();
timer.Interval = TimeSpan.FromMilliseconds(50);
timer.Tick += Timer_Tick;
timer.Start();
}
private void SerialPort_DataReceived(object sender, SerialDataReceivedEventArgs e)
{
// 从串口读取数据
string data = serialPort.ReadLine();
// 解析数据
double value;
if (double.TryParse(data, out value))
{
// 将数据添加到OxyPlot图形中
Dispatcher.Invoke(() =>
{
series.Points.Add(new DataPoint(DateTimeAxis.ToDouble(DateTime.Now), value));
});
}
}
private void Timer_Tick(object sender, EventArgs e)
{
// 更新OxyPlot图形
Dispatcher.Invoke(() =>
{
// 只显示最近的100个数据点
if (series.Points.Count > 100)
{
series.Points.RemoveRange(0, series.Points.Count - 100);
}
// 自动调整X轴范围
if (series.Points.Count > 1)
{
var lastPoint = series.Points[series.Points.Count - 1];
var firstPoint = series.Points[0];
plot.Model.Axes[0].Minimum = DateTimeAxis.ToDouble(firstPoint.X);
plot.Model.Axes[0].Maximum = DateTimeAxis.ToDouble(lastPoint.X);
}
plot.InvalidatePlot(true);
});
}
private void Window_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
// 关闭串口连接
serialPort.Close();
}
}
}
```
在MainWindow.xaml中添加以下XAML代码,用于展示OxyPlot图形:
```xaml
<Window x:Class="SerialPlotter.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Serial Plotter" Height="450" Width="800">
<Grid>
<oxy:Plot x:Name="plot" Margin="10" />
</Grid>
</Window>
```
最后,在App.xaml.cs文件中添加以下代码,以便在程序启动时设置OxyPlot主题:
```csharp
using System.Windows;
using OxyPlot.Wpf;
namespace SerialPlotter
{
public partial class App : Application
{
protected override void OnStartup(StartupEventArgs e)
{
// 设置OxyPlot主题
ThemeManager.SetTheme(Theme.Dark);
base.OnStartup(e);
}
}
}
```
这样就完成了一个动态展示从串口监测数据的图形程序。程序会在启动时打开串口连接,并使用定时器每50毫秒读取一次串口数据,并将数据添加到OxyPlot图形中。图形会自动调整X轴范围,最多显示100个数据点。当程序关闭时,会自动关闭串口连接。
相关推荐
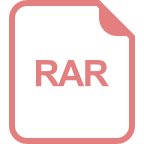














