编写一个python程序,接受一系列空格分隔的单词作为输入,反转字符串中单词的顺序。
时间: 2023-05-27 19:03:43 浏览: 58
```python
s = input("请输入一些单词,单词之间用空格分隔:")
words = s.split()
reversed_words = list(reversed(words))
result = " ".join(reversed_words)
print("反转后的单词顺序为:", result)
```
输入:`hello world my name is John`
输出:`John is name my world hello`
相关问题
python编写一个程序,接受一系列空格分隔的单词作为输入,请你反转字符串中 单词的顺序。
### 回答1:
示例:
输入:hello world
输出:world hello
解释:将输入的字符串中的单词顺序反转,输出反转后的字符串。
Python代码如下:
```python
string = input("请输入一个字符串: ")
# 使用空格分割单词,形成单词列表
words = string.split(" ")
# 反转单词列表
words.reverse()
# 将单词列表转换为字符串
result = " ".join(words)
print(result)
```
### 回答2:
可以使用Python的split()函数将输入的字符串按空格分隔成单词列表,然后再使用reverse()函数将列表中的单词顺序进行反转,最后使用join()函数将反转后的单词列表拼接成字符串。
下面是一个示例程序:
```python
def reverse_words(sentence):
# 将输入的字符串按空格分隔成单词列表
words = sentence.split()
# 反转单词列表
words.reverse()
# 将反转后的单词列表拼接成字符串
reversed_sentence = ' '.join(words)
return reversed_sentence
# 测试程序
input_sentence = input("请输入一系列空格分隔的单词:")
reversed_sentence = reverse_words(input_sentence)
print("反转后的字符串为:", reversed_sentence)
```
运行示例:
```
请输入一系列空格分隔的单词:Hello world, how are you?
反转后的字符串为: you? are how world, Hello
```
以上程序通过split()函数按空格分隔输入的字符串,得到单词列表。然后使用reverse()函数将列表中的单词顺序进行反转。最后使用join()函数将反转后的单词列表拼接成字符串,并将结果输出。
### 回答3:
可以使用split()方法将输入的字符串按空格分隔成一个列表,然后使用[::-1]将列表反转,并使用join()方法将列表中的单词连接起来形成反转后的字符串。
具体代码如下:
```python
# 输入一系列空格分隔的单词
words = input("请输入一系列空格分隔的单词:")
# 将输入的字符串按空格分隔成一个列表
word_list = words.split()
# 将列表反转
reversed_list = word_list[::-1]
# 使用join()方法将列表中的单词连接起来形成反转后的字符串
reversed_string = " ".join(reversed_list)
# 输出反转后的字符串
print("反转后的字符串为:", reversed_string)
```
以上代码可以实现接受一系列空格分隔的单词作为输入,并输出反转后的字符串。
python编写一个程序,接受一系列空格分隔的单词作为输入,请你反转字符串中单词的顺序。
示例:
输入: "hello world"
输出: "world hello"
输入: "I love python"
输出: "python love I"
代码如下:
string = input("请输入一系列空格分隔的单词:")
words = string.split(" ")
words.reverse()
result = " ".join(words)
print("反转后的单词顺序为:", result)
相关推荐
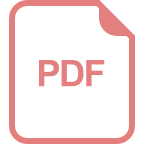
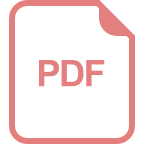












