linux多线程编程实例
时间: 2023-04-30 17:01:54 浏览: 85
Linux多线程编程实例可以使用pthread库进行实现。下面是一个简单的例子:
```
#include <pthread.h>
#include <stdio.h>
void *print_message_function( void *ptr );
main()
{
pthread_t thread1, thread2;
const char *message1 = "Thread 1";
const char *message2 = "Thread 2";
int iret1, iret2;
/* Create independent threads each of which will execute function */
iret1 = pthread_create( &thread1, NULL, print_message_function, (void*) message1);
iret2 = pthread_create( &thread2, NULL, print_message_function, (void*) message2);
/* Wait till threads are complete before main continues. Unless we */
/* wait we run the risk of executing an exit which will terminate */
/* the process and all threads before the threads have completed. */
pthread_join( thread1, NULL);
pthread_join( thread2, NULL);
printf("Thread 1 returns: %d\n",iret1);
printf("Thread 2 returns: %d\n",iret2);
exit(0);
}
void *print_message_function( void *ptr )
{
char *message;
message = (char *) ptr;
printf("%s \n", message);
}
```
在上面的例子中, main() 函数创建了两个线程,分别执行 print_message_function() 函数。pthread_create() 函数用于创建新线程,pthread_join() 用于等待线程结束。
需要注意的是,这个例子是没有考虑线程同步的问题,如果需要进行同步,需要使用pthread库提供的互斥量等工具。
相关推荐
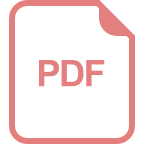














