怎样将int main函数改为void fun
时间: 2023-12-31 17:03:57 浏览: 70
将原来的 `int main()` 改为 `void fun()`,并且去掉 `return` 语句即可。例如:
```c++
#include <iostream>
void fun() {
std::cout << "Hello, world!" << std::endl;
}
int main() {
fun();
return 0;
}
```
改为:
```c++
#include <iostream>
void fun() {
std::cout << "Hello, world!" << std::endl;
}
int main() {
fun();
}
```
注意,`main` 函数是程序的入口,它的返回值表示程序的结束状态,一般来说返回值为 0 表示程序正常结束,非 0 表示程序异常结束。因此在将 `main` 函数改为其他函数时,需要注意函数的返回值是否符合要求。在上面的例子中,我们去掉了 `return` 语句,表示程序正常结束。
相关问题
编写一个函数fun,交换两个数的值,要求使用指针实现。然后再main函数中调用fun函数。fun函数的头部为void fun (int *a,int *b),用C语言
以下是使用指针交换两个数的值的C语言代码:
```c
#include <stdio.h>
void fun(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 10, y = 20;
printf("Before swapping: x = %d, y = %d\n", x, y);
fun(&x, &y);
printf("After swapping: x = %d, y = %d\n", x, y);
return 0;
}
```
输出:
```
Before swapping: x = 10, y = 20
After swapping: x = 20, y = 10
```
#include<stdio.h> int main() { void fun(); int i; for(i=1;i<=5;i++) fun(); return 0; } void fun() { int a=0; ; //定义静态变量 ; //记录函数调用次数 a++; printf("a=%3d,count=%3d\n",a,count);
这段代码存在一些问题,下面是修正后的代码:
```c
#include <stdio.h>
void fun(); // 声明函数
int main() {
int i;
for (i = 1; i <= 5; i++) {
fun();
}
return 0;
}
void fun() {
static int count = 0; // 静态变量,只会初始化一次
int a = 0;
a++;
count++;
printf("a=%3d,count=%3d\n", a, count);
}
```
修正后的代码中,我们声明了函数 `fun()`,并在 `main()` 函数中调用了五次。在 `fun()` 函数中,我们使用了静态变量 `count`,该变量在函数第一次调用时会被初始化为 0,并在每次函数调用时自增 1。同时,我们还定义了局部变量 `a`,每次函数调用时都会被初始化为 0,并自增 1。最后,我们使用 `printf()` 函数输出 `a` 和 `count` 的值。
阅读全文
相关推荐
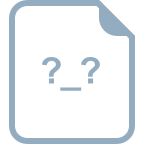
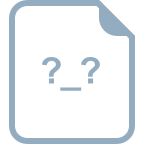
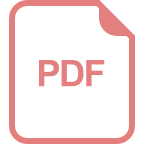














